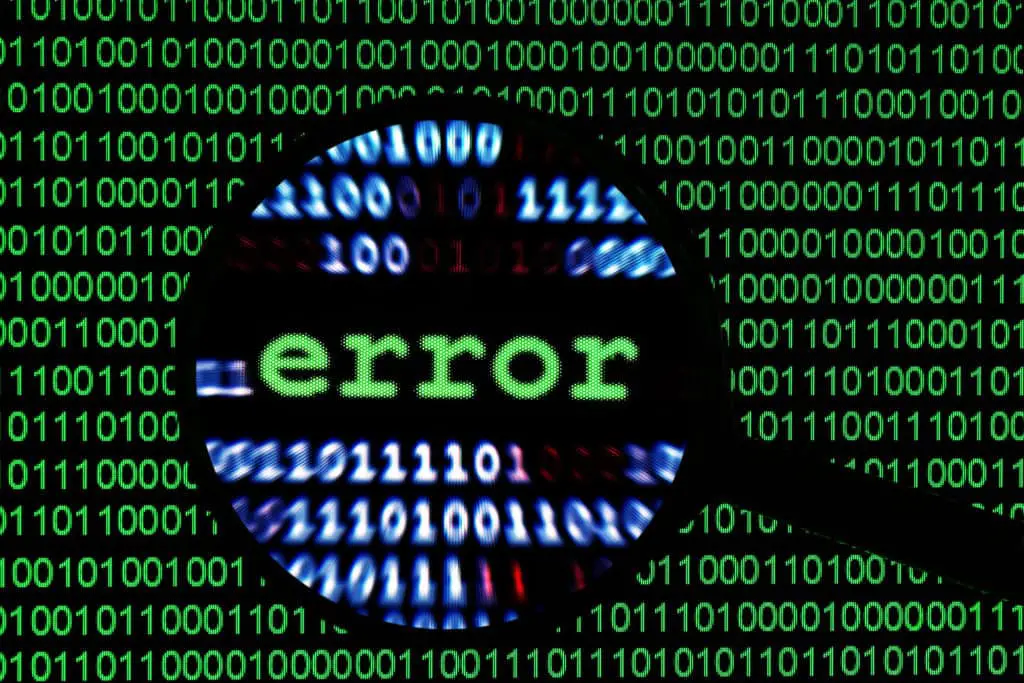
Programming always comes at the cost of errors. Some of them are diabolic and are pretty tricky to deal with, even if you know the position of the error. There are many types of mistakes in a program- one of the most popular errors is the Python IndexError. IndexError refers to incorrect indexing of a variable. If you encounter an IndexError, you have probably given an index to that tuple that does not exist. To rectify it, check the index of the variable, or check the loop range if you are using a loop.
What is a Tuple?
A tuple is a collection of data in a single variable. A tuple can store multiple values within it. The values are separated by commas and are included in the first brackets.
An example of a tuple is as follows:
tup = (‘a’, ‘b’, 12, 16.45, ‘ab’)
As we can see, a tuple may contain anything, from a character and string to a number and float value.
Tuple vs. List
A list is similar to a tuple, but there is one fundamental difference. Here is an example of a list:
lst = [‘a’, ‘b’, 12, 16.45, ‘ab’]
A list also contains multiple values inside it and is bound by third brackets. The primary difference between a list and a tuple is that a list is mutable, but a tuple is not. It means that a list can be modified, but a value in a tuple cannot be modified once it has been created.
Indexing works similarly in lists and tuples. So, if you get the list index out of range, this solution will work for that too.
Indexing
Indexing is the method of calling one of the data in the list or tuple. For example, imagine you are in a queue, and everyone has a numbered token. The token number is the identity of yourself in the queue.
Similarly, data in a list or tuple are numbered based on their positions. The numbering starts from 0. So, the first element in the list has the number 0, the second element has the number 1, etc. Thus, when you want to use an element from a list, you can call it using its positional number, i.e., the index value.
How to Use Index Values
Let’s take an example. We will use the previously mentioned tuple.
tup = (‘a’, ‘b’, 12, 16.45, ‘ab’)
In this tuple, there are five elements in total. Suppose we need to do some work with the fourth element, i.e., the float value 16.45.
In that case, we call this element using its index number. Here is how we can do it.
var = tup[3]
The index number of the fourth element is 3 since indexing starts from 0. So once we write the above line, the variable var gets the value 16.45. tup[3] means to get the fourth value of the tuple ‘tup.’
A similar thing can be done using lists.
Error: Index Out of Range
Index out of range is one of the most challenging errors to solve, and it becomes even more difficult if there are multiple loops in a program.
Let’s understand why this error occurs. We use the same tuple to explain. Suppose we write:
tup = (‘a’, ‘b’, 12, 16.45, ‘ab’)
var = tup[5]
This will fetch an index out of range error. The reason is simple. There are five elements in the tuple. The index of the last element is 4. But var demands the element with index 5. Since no such element is present in the tuple, Python shows this error.
It’s easy enough to fix the problem here. But when things get complicated, the problem arises.
Index Out of Range Error in Loops
tup = (‘a’, ‘b’, 12, 16.45, ‘ab’)
for i in range(10):
print tup[i]
This will also give index out-of-range error. In this case, we use a for loop, and the index is changing every time the for loop is encountered.
The for loop has a range of 0 to 10. So i range from 0 to 10. For the first four times, the loop works perfectly, printing the results, but then, it fails because the situation becomes this: print tup[5], print tup[6], and so on.
The problem is that the error only tells you the line where the problem occurs. It does not tell you why. Therefore, it is up to the programmer to find and debug this error by looking at the range of all the loops used in the program.
How to Avoid Python Error: Index Out of Range
While it seems difficult to find and solve the index out-of-range error, we can avoid this error while writing a program. Here are some ways you can prevent it.
Suppose you need to print all the values in a tuple, but you don’t know the size of the tuple. In this case, if you write:
for i in range(10):
print tup[i]
The program will throw an error (assuming that you have assumed that the tuple size is 10). Hence, assuming a size and using it in the loop is useless.
In this case, we can write:
for i in range(len(tup)):
print tup[i]
Here, len(tup) gives the size of the tuple, i.e., the number of elements present in it. The len(tup) will return 5.
If we use lengths of tuples as a range for loops, there should not be any index out-of-range error. But there are times when we use multiple indexing methods from multiple tuples or lists within a single loop. In that case, it is better to be careful about this error while using indexing. Also, you must not be scared of this error. It can be fixed by the simple method of checking the range of loops so that the index value does not go out of bounds.
Negative Indexing
Giving a negative value in an index is allowed. A negative index value starts counting from the reverse. Unlike positive indexing, which starts from 0, negative indexing starts from 1. Thus, the last value has index -1, while the first value has index –(len(tup)).
To make things simpler, here is a table showing the index values.
Element | First | Second | Third | Fourth |
+ve index value | 0 | 1 | 2 | 3 |
-ve index value | -4 | -3 | -2 | -1 |
From the table, you can understand how indexing works.
Using the tup example,
Element | a | b | 12 | 16.45 | ab |
+ve index value | 0 | 1 | 2 | 3 | 4 |
-ve index value | -5 | -4 | -3 | -2 | -1 |
Hence, negative indexing has a different value from positive indexing. You need to keep this in mind while using negative indexing in loops.
Other Types of Errors
As a bonus, we will discuss some other types of errors programmers encounter often. While Python is easy and fun to work with, some errors need more time to fix than you can expect.
- Invalid Syntax: This is the most common error in python. It happens when you write a syntax wrong. E.g. if you write for i in range (2) instead of for i in range(2), it will produce an error. This happens because the syntax (style of writing) is not perfect.
- Variable is not defined: This happens when you use a variable not previously defined. This mostly occurs due to typo and can be easily fixed by correcting the name of the variable. E.g. if we define tup and then write var = put[5], the program encounters error put is not defined
- Object not callable/subscriptable: This occurs when you do something with a type of object that you are not supposed to. E.g. if we write
integer = 2323
var = integer[3]
This will produce what we call a TypeError. It will show int object is not subscriptable. Sure enough, you cannot treat an integer as a string and break it into parts to extract a single digit.
These are some of the popular troublesome errors Python users face daily. However, it is important to note that having errors does not mean you are bad at programming. In fact, solving programming errors is fun, entertaining, and rewarding!
Conclusion
Programming may be fun, but sometimes errors spring up that can make a coder’s day a nightmare. One such example is the Python IndexError: tuple index out of range. This can also be encountered while using lists. The logic behind this error is pretty simple.
Solving the errors requires you to understand the indexing techniques and range used in loops if any. In case of any errors, a program stops its execution there, so there may be more such errors lying after that line. Lastly, having an idea about positive and negative indexing can be helpful when it comes to solving this error. Big programs have lots of variables using indexing techniques. Hence, a programmer must be careful in using indexes to avoid this error.
Frequently Asked Questions
- How to deal with different errors in Python?
Each error has different a solution. NameError needs you to check the names of the variables. TypeError needs you to check the usage of an element. SyntaxError needs you to correct the wrong syntax in that particular line. - Can Index Error cause other errors in the program?
Each error is dependable on other errors. In general, if you write one wrong line, Python cannot understand the subsequent lines of code. Hence, Python gives errors on those lines too. It is important for you to understand the root of the error and fix it accordingly. - I have a lot of errors in a program? How long will it take me to fix it?
Errors are best not measured using count. This is because one error may produce numerous new errors. Yet, those lines are perfect. E.g., An extra quote may cause Python to think that the remaining program is an endless script. This makes Python prompt more errors than there actually are. Hence, try to fix the genuine errors, and the others will be gone. - How to start debugging a buggy program?
The errors in the program are displayed as the most recent call last. This is the specialty of python traceback, where the console should be read from bottom to top. This is how you should look at the debug console and fix the errors. Hence, the bottom error should be taken care of first. - Which one is more advantageous, a tuple or a list?
It depends whether a tuple or a list will be more appropriate. A tuple and a list are two similar variables that store elements in them. Both can be used similarly, except that a value in a tuple cannot be modified. Whenever python wants to produce a list of elements, it uses a tuple. You can easily convert a tuple into a list with some lines of code. A list is the best choice when you are working on it, but a tuple is preferred when you are using it as the output. - Does negative indexing give index out-of-range error?
Negative indexing is used to view the list in reverse, and should not encounter any error. However, there are some compilers where the use of the negative index is not permitted. In such cases, you may encounter errors. If you want to access the last element of a tuple without the use of a negative index, you can write var = tup[len(tup)-1] . This will fetch the last element of the tuple. Decreasing 1 from the length is important because positive indexing starts from 0, but len(tup) will return the number of elements in the tuple. - What is the difference between (1,2) and (‘1’, ‘2’)?
(1,2) is a tuple that contains two integers, 1 and 2. (‘1’, ‘2’) is a tuple that contains two strings, ‘1’ and ‘2’. The strings cannot be used as integers and will have to be converted. Similarly, integers cannot be used as strings and will have to be converted.- To convert a string to an integer, we use int(), e.g. int(‘2’)
- To convert an integer to a string, we use str(), e.g. str(2)