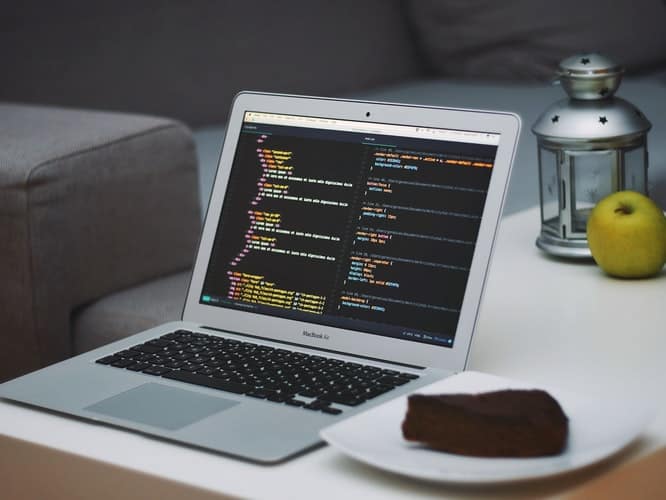
We will discuss Python Syntax Error here. A lot of programmers complain that whenever they use the ‘Return’ statement, they get an output similar to this:

If you’re reading this article, you’re probably having the same issue and needing a solution about PYTHON SYNTAX ERROR: ‘RETURN OUTSIDE FUNCTION’ PROBLEM. You’ve come to the right place. Trust me. It’s really easy: ensure that the return statement is used only within a function and not after the function. There are two major ways that this rule might be broken, leading to the fatal ‘SyntaxError.’ I’ll be pointing out these ditches, but I’d like to explain the ‘return before I do’ statement a bit, first.
Outline:
- What Python SyntaxErrors are
- How the ‘Return’ statement works.
- Python SyntaxError: Return Outside Function
- When the ‘Return’ Statement is used outside a function.
- When the ‘Return’ statement is used within a loop.
- Conclusion: It’s all about Indentation.
What Python Syntax Errors Are:
The information in this section is important because it will help you understand the principle behind the ‘Return Outside Function’ error.
Python Syntax Errors are the most basic errors. You can think of them like this: imagine you’re new to an Asian country and you’re just learning to speak their language. One day you’re talking to some Asians, you’re doing well until you mispronounce a word, and all the indigenes around you become confused. That one word you said wrong has broken the communication link, and they can no longer understand you.
It’s the same thing for Syntax errors. They occur when you miswrite, misspell or mispresent a statement in a program. Wrong indentation and incorrect arguments (parameters) could also lead to a syntax error. A syntax error is when Python is unable to understand a piece of code due to any of the reasons stated above. Codes with syntax errors can’t be executed in any way, so you have to find the error’s location and correct it before your program can run right. I already feel sorry for you if the code with the error is a long and complex one. On the bright side, IDLE (Integrated Development Environment, an optional part of the python packaging) can help you point out and correct the errors in your code.
That said, let’s talk more about the ‘Return’ statement and how it works.
How The Return Statement Works:
Look at the line of code below. The Function is contained within a general scope. This is the way it should be. A function is usually defined like this:

For those who are new to this, ‘Def’ is used as a keyword for defining a function. You can decide the Name of the Function. The Argument can be thought of as the variables that will hold the values that your program will use.
The ‘Return’ statement acts more like a portal, allowing the transfer of values from a function to another function or to the general scope where it can be used to solve other problems. The ‘return’ statement is to come at the end of a code block in a function. Remember the General scope I mentioned earlier? It is capable of holding various functions that may depend on each other to achieve an aim. For these functions to relate to each other, the keyword ‘Return’ is used. This way, results obtained in previous functions can be used to solve problems in another function. I guess this circle diagram will help you understand the general scope and function ideology better.
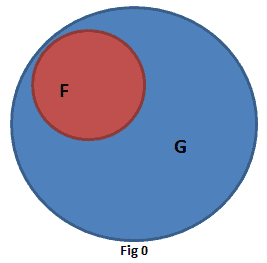
Here F is a function within a general scope, G. What happens within this function also affects the general scope.
Let’s now use a real program to illustrate my point. Take a look at the program below:
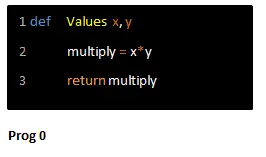
In this function defined above, we want to multiply two numbers. Say, for example, in the general scope. We want to use the function to find the product of 10 and 12. The ‘Return’ statement in this function allows you to call the function in the general scope.
A we have to do is:
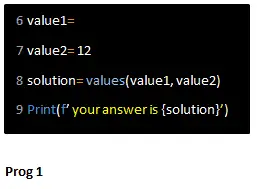
With this, the properties of the function will affect the values provided in the general scope. That’s all.
Using the ‘Return’ function is easy and straightforward. Usually, you shouldn’t get into any problems as long as you use it the right way- only within a function.
Python Syntax Error: Return Outside Function:
A lot of newbies at this sequence make a mistake with the ‘Return’ statement. The ‘Return’ statement must only be used within a Function. It can’t be used within a loop either. Most times, the only thing that makes a difference is the Indentation. The ‘Return’ statement’s indentation will determine whether it’s in a function or within a loop. Let’s look further into these scenarios in the subsections below, shall we?
When The ‘Return’ Statement Is Used Outside A Function:
Let me begin this explanation using something that everyone, whether expert or amateur, can relate to.
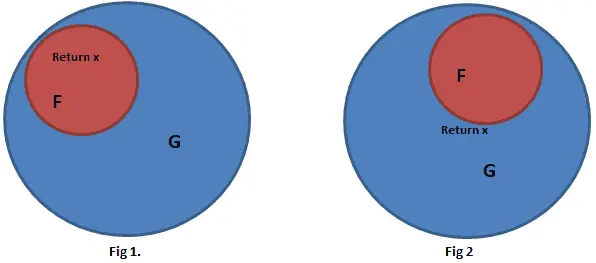
Remember this diagram from above? You might want to notice the position of ‘Return x’ within the circle tagged F. As long as it is inside this circle, your program will run effectively. However, as in Fig 2, once ‘Return x’ is outside the circle, your program is bound to crash.
Now, let’s rewrite our code above:
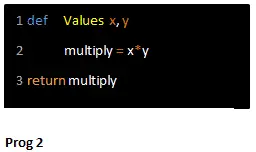
Pay close attention to the position of the ‘Return’ statement in this new program and the one above. Did you notice that the ‘Return’ keyword in this new program is indented less to the right than in the previous program? It looks like a small difference. In fact, it seems so inconsequential, and it could hardly be noticed most times.
However, this small detail is capable of ruining your whole program.
What this new indentation means is that the ‘Return’ statement is no longer within the Function. Because the ‘Return’ statement was tailored to toggle variables between a function and the main program, once the ‘Return’ statement is outside a Function as in the above program, it no longer serves a purpose. It no longer has any connection with the variables within the above function, even though it is attached to a variable within the function. You can think of it as a young man holding the picture of his crush. He may have her picture with him, but as long as he isn’t in the same room with her, he can’t touch her.
So just ensure that your ‘Return’ statement is correctly indented: one step to the right of the Def function, not on the same line with it, and your program will be just fine. If not, rather than use the ‘return’ statement, use the ‘Print’ statement.
When The ‘Return’ Statement Is Used Within A Loop Or A Condition Statement:
Another common mistake programmers make to use the ‘Return’ statement within a loop. A loop is a statement that allows an instruction to be carried out repeatedly. Usually, the statements ‘While’ and ‘For’ are used for looping. The ‘For’ loop repeats the instructions for the number of times you predetermine, while the ‘While’ loop will repeat the code as long as a certain condition is met. See the below program for example.
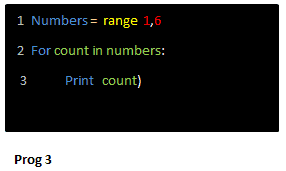
You can see that as long as the range of numbers is less than 6, the program will continue to print a value (increasing digits from one to six). That’s what a ‘For’ loop does.
On the other hand, conditional statements serve as testers under certain conditions. They use the ‘if,’ ‘then,’ ‘else, and ‘elif’ statements. If a certain condition is met, then a certain output will be given. If the reverse is the case, then another predetermined output will be given. For example, look at this program I took from careerkarma.com below:
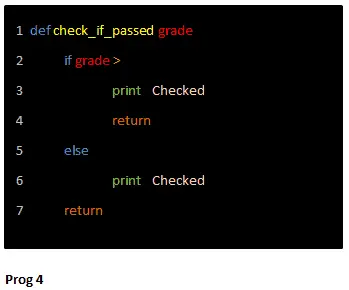
Here we can see that if the grade is greater than 50, then the program will print ‘checked’ and return True; however, if the grade is less, the program will print ‘checked and return false. We could also call it a conditional statement.
What does this have to do with the ‘return outside function’ syntax error, you might ask. And I’ll tell you: firstly, under no circumstance should your ‘return’ be indented within a loop.
As usual, before we look at a complex program, I’d want us to look at my circle diagram again:
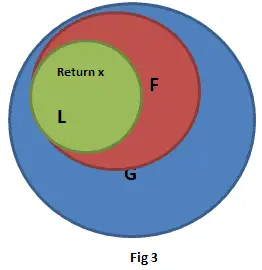
You can see that within our general scope circle, we have our function circle and, within the Function circle, we have another small L circle. That L stands for Loop. By now, we all know that under no circumstance should your ‘return x’ be outside the F-marked circle (at least, you should if you have been paying attention so far). However, what if the ‘Return x’ statement is within the function and the loop?
The answer is simple: it separates your ‘Return x’ from the function and renders your program useless. Python will be more than glad to give you the response:

Once again, the principle lies in the indentation. Look at the program below.
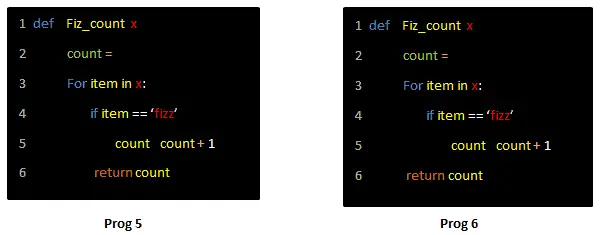
Notice in Prog 5 that the ‘return’ statement is indented one step further to the right from the ‘For’ statement? This structure means that the ‘return’ statement is within the loop and will not be considered a part of the function. Running this program will lead to a Return Outside Function error.
To solve this, ensure your ‘return’ statement is indented to be on the same line as the ‘For’ statement as in Prog 6. This way, the ‘return’ statement is outside the loop and is properly represented within the program.
The conditional statement follows a slightly different rule. Usually, you can use the ‘return’ statement within a conditional block; however, the game changes when dealing with a ‘True’ ‘False’ situation, popularly known as Boolean. Let’s look at Prog 4 from above.
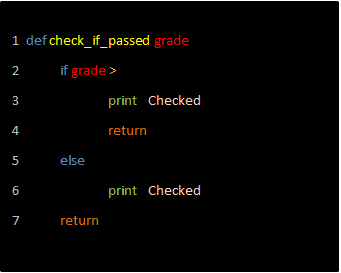
You might have noticed that the first ‘return’ statement is within the ‘if’ block while the other is outside the else block. This is the right arrangement. I know it looks tricky, but you need to pattern your program this way if you hope for a correct result.
Conclusion: It’s All About Indentation:
Indentation is a very important factor in writing any program in Python. As I mentioned above, most Python errors are simply because of something wrongly written or wrongly indented. Indentation accounts for a major number of errors on python, so you must be careful about it.
The position of any function or statement influences its usability. In fact, wrongly indenting a statement can alter its purpose and even change your program’s output. Take note of these little things when you’re running your program.
So let’s try one more program then, shall we? Can you tell me what is wrong with this program?
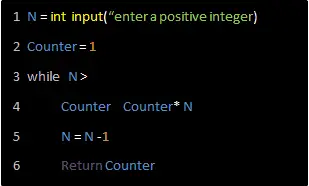
If your answer was that no function was defined in the first place, thus rendering the Return statement void, then you said the right thing. Kudos to you.
If you ever have a problem using the ‘return’ statement, it’s most likely due to one of the two problems stated above. However, if you feel your program has been well written and you still have error messages as output, do well to contact me using the comment box.
I’m sure I have the solution. See you in a later blog.
Also read Is Python a Scripting Language or Programming Language?