Introduction
Python is a widely used and known high-level programming language. The language is a recent discovery and has been used commonly in the software industry for designing apps and websites. This is because it is an easy-to-learn language and has a wide range of libraries that are in-built and can be of great use. In Python, most of the job gets easier when compared to the other programming languages, like declaring the type of variable or type conversion. Let us know ‘Python Compare Strings’.
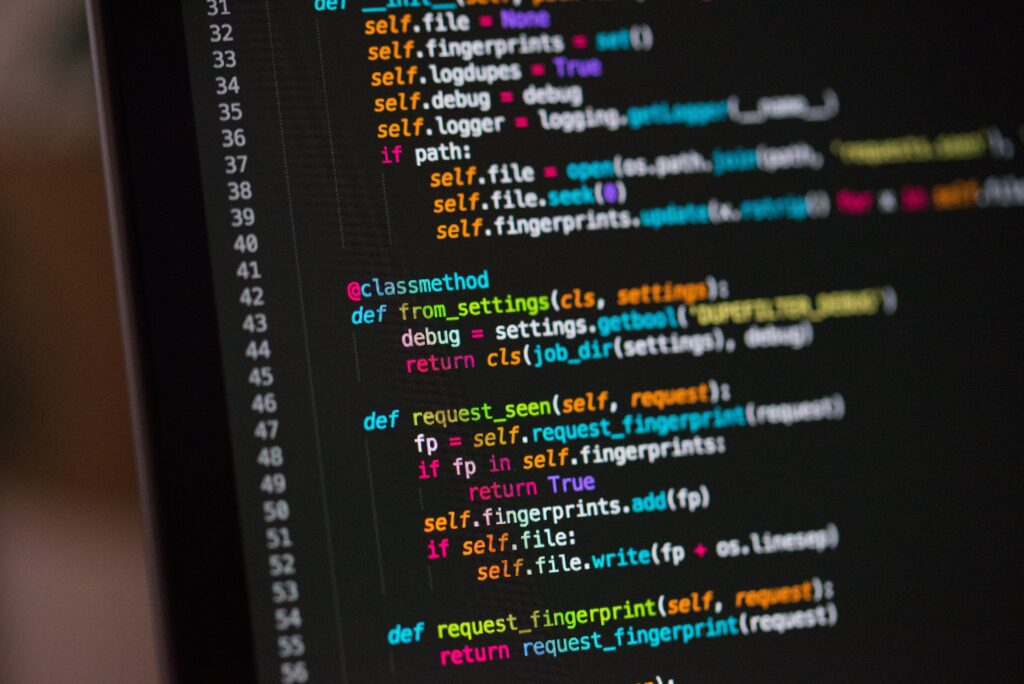
Python Compare Strings
Compare strings in Python has a variety of applications in most of the sectors where Python is used. It is not only numerals that are to be compared often but strings too. Comparisons of strings are done with the == and != operators. They compare the two given strings and return either True or False. There are other such comparison operators in Python that help in the comparison of strings.
In this article, we will see more about the comparison operators available in Python and how to use them to compare strings.
Strings equal to and not equal to
The == and the != operators, also called the is equal to and is not equal to operators respectively, are used in Python to compare strings and return either True or False. The == operator compares the two given strings and if they are equal, it returns True. if the two strings are not equal, the output returned is False. The total opposite is what is given from the != operator. If the given two strings are found to be equal, the output returned is False. On the other hand, if the two given strings are not equal to each other, the value True is returned.
The == operator, for instance, can be made use of to compare your entered password to the one you have already set and if the two passwords are the same, you will be allowed to access your site. It is important to note that strings in Python are case-sensitive. This means that ‘hello world’ is not the same as ‘Hello world’. In the following code, let us see what happens.
#string comparison
#this is using the == operator
string1=’hello world, this is me.’
string2=’hello world, this is me.’
if string1==string2:
print(“The two strings are equal”)
else:
print(“The two strings are not equal”)
OUTPUT
The two strings are equal.
Another example,
#string comparison
#this is using the == operator
string1=’Hello world, this is me.’
string2=’hello world, this is me.’
if string1==string2:
print(“The two strings are equal”)
else:
print(“The two strings are not equal”)
OUTPUT
The two strings are not equal
The same can be applied to the not equal to operator.
#string comparison
#this is using the != operator
string1=’Hello world, this is me.’
string2=’hello world, this is me.’
if string1!= string2:
print(“The two strings are not equal”)
else:
print(“The two strings are equal”)
OUTPUT
The two strings are not equal
#string comparison
#this is using the != operator
string1=’hello world, this is me.’
string2=’hello world, this is me.’
if string1!= string2:
print(“The two strings are not equal”)
else:
print(“The two strings are equal”)
OUTPUT
The two strings are equal
Python is and is not operator
These two operators are also comparison operators and in most cases, they can act as a substitute for the other == and != operators. You might prefer using the is and is not operator when you want to compare the object ids of the given strings rather than comparing the actual value as the other comparison operators do. The is and is not operators generally occupy less space than their counterparts. Let us use this in a code.
Say you want to compare the marks of two different students and check if they are equal or not.
#string comparison
#this is using the is operator
mark1=’462’
mark2=’462’
if mark1 is mark2:
print(“The two students scored the same marks”)
else:
print(“The two students have not scored the same marks”)
OUTPUT
The two students scored the same marks
Let us use the same code with the is not operator and see what happens
#string comparison
#this is using the is not operator
mark1=’682′
mark2=’682′
if mark1 is not mark2:
print(“The two students have not scored the same amount of marks”)
else:
print(“The two students scored the same marks”)
OUTPUT
The two students scored the same marks
Other comparison operators in Python
There are other such comparison operators in Python like
< – less than
> – greater than
<= – less than or equal to
>= – greater than or equal to
These operators can be used in the same way as the above-mentioned operators were used. But let us try them out in code.
#string comparison
#this is using the comparison operators
name1=’Robert’
name2=’William’
if name1<name2:
print(“Out of the two given names,”,name1,”comes first in alphabetical order”)
else:
print(“Out of the two given names, “,name2,”comes first in alphabetical order”)
OUTPUT
Out of the two names, Robert comes first in alphabetical order
This program compares two strings and returns the one that comes first in alphabetical order.
The same program when used with the greater than operator will give exactly the opposite result. On using the lesser than or equal to operator, we can compare two strings.
#string comparison
#this is using the comparison operators
num1=’64829′
num2=’46282′
if num1<=num2:
print(“Out of the two given numbers,”,num1,”comes first”)
else:
print(“Out of the two given numbers, “,num2,”comes first”)
OUTPUT
Out of the two given numbers, 46282 comes first
The same logic can be applied with the greater than or equal to operator and the result will be the exact opposite of what is returned in the above code.
Using integers or floats for comparing numbers is indeed a better option since all of these comparison operators are applicable for those as well, but the above-mentioned examples are merely examples, and you can substitute the numbers with words or letters to see how the code would work.
Conclusion
Python is, with proper reason, one of the easiest code languages to learn. There are a lot of options available for the same operation and you can make use of these to do the same task in different ways. Comparing strings is also easy when you understand the mechanism of the algorithm and the flow of the code. Comparing strings is very useful in a variety of sectors, from passwords to comparing items in huge databases.
FAQs
- Is it possible to compare more than two strings at the same time?
It is indeed possible to compare more than three string at the same time and it is pretty easy too.
- Is it easy to compare strings in Python?
As seen above, the comparison of strings is very easy in Python.