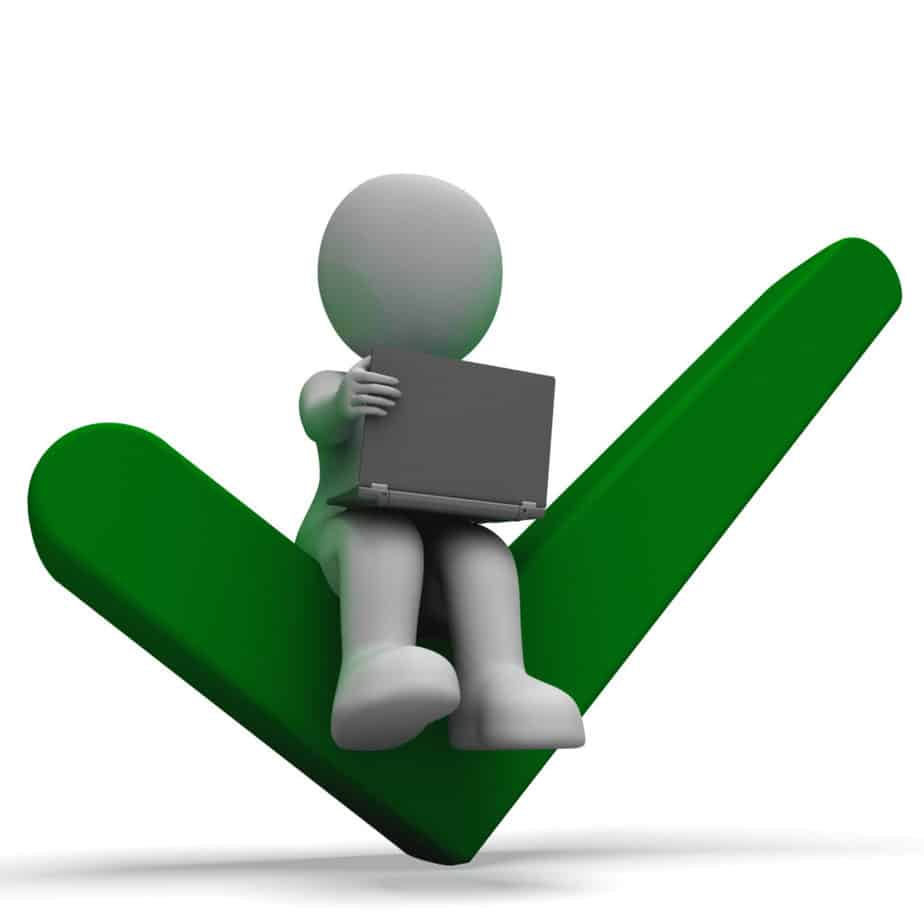
In this article. we will see the Python NameError and Name is Not Defined solution easily.
Introduction
Programming always gives you errors, and many a time, the errors are caused due to our mistakes. When you type quickly, typos are bound to occur, and they cause a lot of errors that flash in unexpectedly. Such an error is the NameError, where Python fails to understand some data. NameErrors are caused due to typos or wrong declarations.
A Python program shows NameError when Python fails to understand the name of a variable or library. This can be solved by carefully checking the program for typos and variable names. Python says that a name is not defined, which means that whatever you have entered in that line does not exist in the program. Variables should be declared before usage.
Python NameError
Python has a lot of error messages popping up when you run your code for the first time (most of the time). While several errors are caused due to logical errors, sometimes simple typos cause problems. Python cannot fix any errors on its own, so these errors also pile up the console in red color.
Python NameError is the error easiest to solve because it does not happen due to any logic issues. NameError: name is not defined error is caused when Python, saying simply, does not understand what you have written. While in most cases, it is just a typo, sometimes, things may become complex. In this article, we will discuss different reasons for getting the NameError error message.
Causes of Python NameError
As previously mentioned, the NameError problem occurs when you write something Python does not understand. There can be many reasons for this error. Here are some of them:
- Typo in naming a variable
- Declaring a local variable and using it outside the function
- Not declaring a variable
- Declaring a variable after calling it
We will discuss these error reasons one by one, and this will solve the problem with your program if you are encountering this error message. Don’t worry – we will also provide examples for better understanding.
Typo In Naming a Variable
This is the most common reason for getting the Python NameError: the name is not a defined message. Consider the below example:
var = 2
print (vary)
This will throw an error “vary is not defined”. You can understand why – the name of the variable is var. While printing the variable, there is a different name used, mostly occurring due to a typo.
This can be easily resolved by checking the variable name while declaration and making sure the same name is used while calling it.
Using Local Variable Outside the Function
A second probable reason for the error is that you have declared a variable out of scope. Before jumping into the solution, let’s see what declaring variables in scope exactly means.
Whenever you declare a variable, you either declare it during the start of the program (global declaration) or inside a function (local declaration). When you declare a variable globally, you can use it everywhere in the program. But if you declare the variable locally, you will not be able to use the variable outside the same function. Let’s take an example:
var = 23
def Print():
x = 24
print (var)
print (x)
The above program will show the NameError: x is not defined. Why? Because we have called x outside the Print function, where x is defined. This is called calling out of scope.
To solve this problem, ensure that you have called all the variables in scope. If not, you can either globally declare the variables (like var in the program), or change the statement to call the variable from the function.
Not Declaring a Variable
Sometimes, we call a variable by mistake without declaring it. See the below example:
x = 2
y = 3
print (x+y+z)
Here, we can see that z is not defined. Hence, the program throws the error. Whenever the error pops up, check whether you have defined the variable before using it.
Note that Python supports dynamic declaration. This means that we can directly write x = 2 instead of declaring the type first.
In C, we write:
int x = 2; or int x; x = 2;
In Python, we do not have to declare the type of the variable, it does that for you.
Since Python runs from top to bottom, the variables need to be declared first before usage. Otherwise, Python has no idea what you are talking about.
Declaring Variables at the Wrong Place
As said before, Python reads from above. Hence, whenever it has to do some operations, it relies on the data it has read, i.e., the data before that line. Hence, it is necessary to declare the variables before you use them.
print (var)
var = 3
This shows the NameError: var is not defined. You can understand why – the variable has been declared after it has been used, so Python gives an error in the first line itself.
You should also remember that Python stops program execution whenever it encounters an error. This can be taken care of using a try-except feature, but that is a separate discussion. Hence, anything written in the lines below the wrong line will not be executed.
Wrong Library Names
Another mistake leading to this error is importing the wrong library or calling it wrongly. Let’s take an example:
import collections
…
N = collectons.permutation(…)
Here, spelling the name of the library wrong results in the NameError. Also, consider this example:
from collections import permutation
…
N = collections.permutation(…)
This also produces a NameError because you have not imported collections but only permutations. So, Python does not know what collections are. Write N = permutation(…) to fix the problem.
A third example:
Import collections
…
N = permutation(…)
This also produces NameError because you have imported the whole library collections and not just permutation. Hence, you have to guide Python (i.e., write collections.permutation(…)) in choosing the function you want to use.
Other mistakes leading to this error
Python is a simple language, but people still make crazy mistakes. Some mistakes leading to this error include:
- Indentation errors
- Quote errors
- Syntax errors
Needless to say, these errors are self-explanatory, and if you are a programmer guy, you know what these things are. There are many IDEs that give different reactions towards a written line, so be careful while you code. Sometimes, an error in the previous line may also cause issues. In that case, check some lines immediately above the error-tagged line.
Many IDEs give red marks under variables that are not declared. Think those like the red-marked words in a Word document. Green marks are also provided occasionally, which means there is an error regarding the syntax. Indentation errors are also marked in red, showing that there are unwanted indentations before a line.
Ultimate guide to solve this error
- Check the variable name, both while declaration and using it
- Check the scope of the variable, make sure it is in scope
- Check the place of declaration of the variable, make sure it is declared prior to usage
- Check for indentation errors or quotation errors in the line
- Check for errors in the previous line that may cause such an issue
- Check for red and green marks, if your IDE provides them
- Check for the library names and make sure you have the right library imported
Conclusion
Python NameError is caused when Python does not understand the name of a variable. While most of the time, the reason is a simple typo, sometimes things get complicated.
The above guide is enough to help you resolve the issue. Checking the variable and library names is the remedy for this error. Sometimes, NameError is caused due to indentation errors or syntax errors. In this case, the real error may not be in that same line but the line above.
Since Python reads from above, it is necessary to declare a variable before using it. Python also supports the dynamic declaration that helps the user to declare and use the variable in the same line.
NameError is quite common when programmers code, and it is one of the easiest of errors to solve. However, in times of coding big problems, finding the declaration statement and checking the import statements may be a hassle.
Frequently Asked Questions
Q1) How is the Python NameError displayed in the console?
A: The error is generally displayed when you are trying to run a program. The line number is displayed beside the error message. The real reason for the error message may not be the same line, so make sure you check the line above too.
Q2) How to know what problem has caused this issue?
A: It is not possible to say the real reason for the error by just reading the error message. To understand the reason, you have to check the program thoroughly. It is possible that one typo has ruined your whole program.
Q3) I cannot find the source of the problem. What should I do?
A: Follow the red and green lines, if your IDE provides them. There are online debuggers that may help you to solve the problem. But since this error message is shown due to simple reasons, you can fix it yourself. Just follow the points in the article and make sure you check every line where you have used and declared the error variable.
Q4) My print statement gives the NameError error, although I have written the variable name correctly. What should I do?
A: The reason your print statement provides an error may be because you are trying to print a single word, and you have forgotten to provide quotes before and after it. A print statement requires a variable or a string. Strings should be within quotes, otherwise, Python thinks it is a variable.
Q5) The variable name is shown in red in my IDE. Is that because of a NameError?
A: No, the red color does not mean any error. Some IDEs show variable names in red. The error message will not be shown until you run the program. The message will be displayed below in the console. The red underline in the program may mean errors. The coloration of the script helps you to make sure your writing matches what you are thinking. For example, a red color will ensure that Python really understands your variable as a variable.
Q6) What are some other errors that are similar to NameError?
A: There are many errors like this. Syntax errors are caused due to problems with the syntax. Indentation errors are caused due to the wrong indentation. These are not logical errors and do not require changes in the program logic to resolve. This is the reason these are so easy to rectify.
Q7) How is NameError different in other programming languages?
A: The NameError may be written differently in other programming languages. The key is the variable is not a defined statement. It will help you know that they are asking you to correct the name of the variable.
Q8) Are there any naming conventions that cause NameError?
A: No, naming conventions do not cause the NameError. However, naming a variable similar to a predefined object gives you a separate error, which depends on what you write. For example, naming a variable list or pass causes errors to pop up. The reason is that Python thinks you have called the object instead of thinking of it as a name of a variable. The color of the script will tell you whether Python takes the variable name as a valid name of a variable.
Q9) How can I reduce the chances of getting a NameError issue?
A: The only way to reduce chances is to be cautious about typos. Some IDEs provide you the options of Tab-complete or Enter-complete, which can be helpful. They are shortcuts for writing the variable name. Hitting enter will write the variable name when required if you enter the first few letters of it. This way, you cannot encounter an error because Python takes the name from the previous lines. Hence, the same variable name is being used throughout the program.