Introduction
The sliding window concept refers to a technique used to process and analyze data streams, where the data is divided into fixed-size windows that are moved or “slid” over the data set. The goal of the sliding window technique is to efficiently compute certain properties or statistics of the data within each window, such as the maximum value, minimum value, average value, etc.
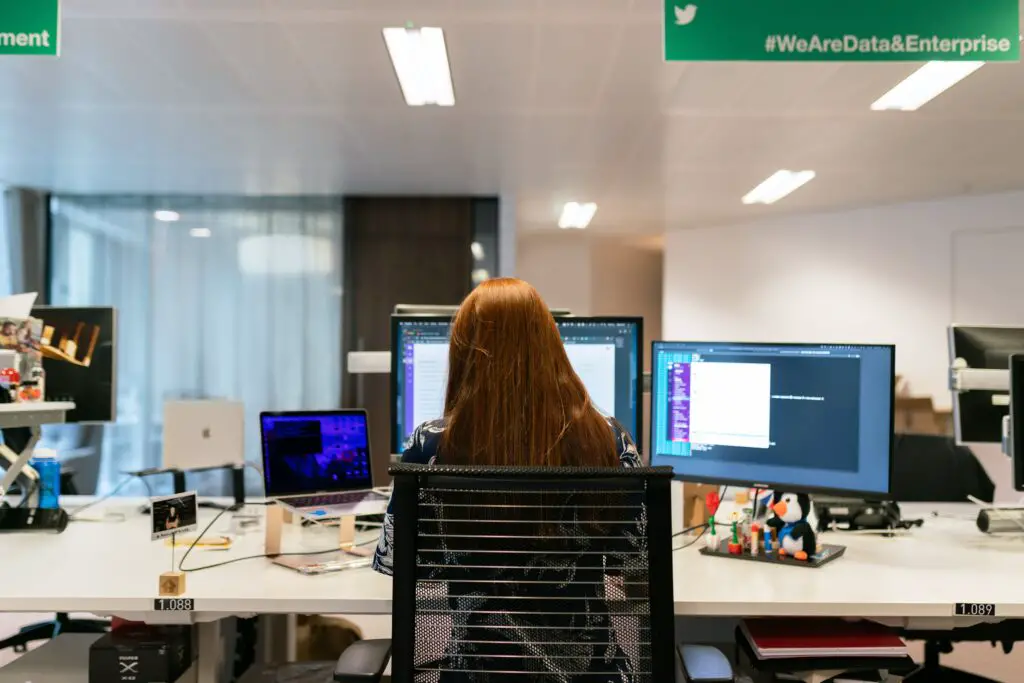
Sliding Window Maximum
In the context of computing maximum values, the sliding window technique is used to find the maximum value within a fixed-size window of data. This can be useful in a variety of applications, such as image processing, signal processing, and financial analysis, where it is necessary to identify and track local maximums or peaks in the data.
For example, in image processing, the sliding window maximum algorithm can be used to find the brightest pixels in an image, while in financial analysis, it can be used to identify the highest price of a stock within a given time period.The sliding window maximum algorithm works by maintaining a data structure, such as a deque or a priority queue, that keeps track of the maximum value within the current window. As the window is moved over the data set, elements are added and removed from the data structure to reflect the current window. The maximum value is then extracted from the data structure as the window moves along.
Different algorithms for solving the sliding window maximum problem
There are several algorithms for solving the sliding window maximum problem, including:
- Deque Method: This approach uses a double-ended queue (deque) to store the indices of the elements within the current window. The deque is maintained such that the elements in the front of the deque are always in decreasing order, while the elements at the back are in increasing order. As the window is moved over the data set, elements are added and removed from the deque to reflect the current window. The maximum value is then extracted from the front of the deque.
- Dynamic Programming: This approach uses dynamic programming techniques to compute the maximum value within the current window. One way to do this is to precompute the maximum value for each subwindow and then use these values to compute the maximum value for the current window.
- Heap Method: This approach uses a max-heap data structure to maintain the elements within the current window. As the window is moved over the data set, elements are added and removed from the heap to reflect the current window. The maximum value is then extracted from the top of the heap.
- Monotonic Queue: This approach uses a queue that maintains a monotonic order of elements. In this approach, the elements are added to the queue and if an element is smaller than the last element, it will be removed from the queue. The maximum value will be always on the front of the queue.
Each algorithm has its own advantages and disadvantages in terms of time and space complexity, ease of implementation, and performance. The choice of algorithm will depend on the specific requirements of the application and the trade-offs that are acceptable.
Applications of the sliding window maximum in various fields
The sliding window maximum algorithm has a wide range of applications in various fields, such as:
- Image Processing: The algorithm can be used to identify and track local maximums or peaks in an image, such as the brightest pixels in a video or the highest intensity values in a medical scan. For example, in computer vision, the sliding window technique is used to detect objects in an image by sliding a window over the image and computing the maximum value within each window.
- Signal Processing: The algorithm can be used to analyze signals such as audio, speech, or sensor data. For example, in speech recognition, the sliding window technique is used to segment an audio signal into overlapping frames and compute the maximum value within each frame to identify speech patterns.
- Financial Analysis: The algorithm can be used to analyze financial time-series data, such as stock prices, and identify local maximums or peaks in the data. For example, the sliding window technique can be used to detect the highest price of a stock within a given time period.
- Bioinformatics: The sliding window technique is used in bioinformatics to analyze genomic data such as DNA or RNA sequences. The algorithm can be used to identify patterns or motifs within the sequence, such as the location of a certain gene or the presence of a particular mutation.
- Natural Language Processing : In NLP, the sliding window technique is used to process sequences of words in a text. The algorithm can be used to identify patterns or motifs of words, such as the presence of specific phrases or the sentiment of a text.
These are some of the examples of how sliding window maximum algorithm is used in different fields. However, the algorithm can be applied in other fields as well depending on the requirements of the problem.
Comparison of the time and space complexity of different sliding window maximum algorithms
The time and space complexity of different sliding window maximum algorithms can vary depending on the specific algorithm and data structure used. Here is a general comparison of the time and space complexity of some common algorithms:
- Deque Method: The time complexity of the deque method is O(n) where n is the number of elements in the data set. This is because each element is processed once as the window is moved over the data set. The space complexity is also O(n) as the deque stores the indices of the elements within the current window.
- Dynamic Programming: The time complexity of the dynamic programming approach is O(n^2) where n is the number of elements in the data set. This is because the maximum value for each subwindow must be computed. The space complexity is also O(n^2) as a table is used to store the maximum value for each subwindow.
- Heap Method: The time complexity of the heap method is O(n log k) where n is the number of elements in the data set and k is the size of the window. This is because elements are added and removed from the heap as the window is moved over the data set, and the maximum value is extracted from the top of the heap. The space complexity is also O(k) as the heap stores the elements within the current window.
- Monotonic Queue: The time complexity of the Monotonic Queue approach is O(n) where n is the number of elements in the data set. This is because each element is processed once as the window is moved over the data set. The space complexity is also O(k) as the queue stores the elements within the current window.
It is important to note that these are just general complexities, the actual complexities might vary depending on the implementation and specific use case. Also, the choice of algorithm will depend on the specific requirements of the application and the trade-offs that are acceptable.
Examples and implementation details of the sliding window maximum algorithm in various programming languages
The sliding window maximum algorithm can be implemented in various programming languages such as C++, Java, Python, etc. Here is an example of how the deque method can be implemented in Python:
from collections import deque
def sliding_window_maximum(arr, k):
n = len(arr)
dq = deque()
result = []
# Process first k elements
for i in range(k):
while dq and arr[i] >= arr[dq[-1]]:
dq.pop()
dq.append(i)
# Process the rest of the elements
for i in range(k, n):
result.append(arr[dq[0]])
# Remove out of window elements
while dq and dq[0] <= i-k:
dq.popleft()
# Remove smaller elements in k range as they are useless
while dq and arr[i] >= arr[dq[-1]]:
dq.pop()
dq.append(i)
result.append(arr[dq[0]])
return result
# Example usage
arr = [1, 3, -1, -3, 5, 3, 6, 7]
k = 3
print(sliding_window_maximum(arr, k))
# Output: [3, 3, 5, 5, 6, 7]
This implementation uses a deque to store the indices of the elements within the current window. The deque is maintained such that the elements in the front of the deque are always in decreasing order, while the elements at the back are in increasing order. As the window is moved over the data set, elements are added and removed from the deque to reflect the current window. The maximum value is then extracted from the front of the deque and added to the result array.
Similarly, you can implement sliding window maximum using other algorithms like dynamic programming, heap, monotonic queue in Python with the help of appropriate data structures.
It is important to note that the implementation may vary depending on the programming language and the specific requirements of the application.
It is always a good idea to test the performance of the implementation with different inputs and optimize it accordingly.
A discussion of trade-offs and limitations of the sliding window maximum algorithm
The sliding window maximum algorithm is a powerful technique that can be used to efficiently process and analyze data streams, but it also has some trade-offs and limitations. One trade-off of the algorithm is that it can be computationally expensive for large data sets, especially if using an algorithm like dynamic programming that has a high time complexity. This can be mitigated by using more efficient algorithms like the deque method or the heap method.
Another trade-off is that the sliding window maximum algorithm can be sensitive to the size of the window. A larger window size may lead to a smoother result but may miss some smaller peaks or local maximums, while a smaller window size may lead to more accurate results but may also introduce more noise. Additionally, the sliding window maximum algorithm assumes that the data is stationary, meaning that the statistical properties of the data do not change over time. However, if the data is non-stationary, this assumption may not hold, and the algorithm may produce inaccurate results.
The sliding window maximum algorithm also assumes that the data is uniformly sampled. For example, if the data is sampled at different frequencies, the algorithm may produce inaccurate results. Another limitation of the sliding window maximum algorithm is that it assumes that the window size is known in advance. If the size of the window is not known, it may be necessary to use a more sophisticated algorithm that can adapt the window size to the data.
In summary, the sliding window maximum algorithm is a powerful technique that can be used to efficiently process and analyze data streams, but it also has some trade-offs and limitations. It is important to consider these trade-offs and limitations when using the algorithm and to choose the appropriate algorithm and window size for a specific application.
Conclusion
In conclusion, the sliding window maximum algorithm is a powerful technique used to efficiently process and analyze data streams. The algorithm is used to compute the maximum value within a fixed-size window of data, which is useful in a wide range of applications such as image processing, signal processing, financial analysis, bioinformatics, and Natural Language Processing. There are various algorithms available for solving the sliding window maximum problem, such as the deque method, dynamic programming, heap method, and Monotonic Queue.
Each algorithm has its own advantages and disadvantages in terms of time and space complexity, ease of implementation, and performance. The choice of algorithm will depend on the specific requirements of the application and the trade-offs that are acceptable. The article provided an overview of sliding window maximum, its subtopics, applications, examples and implementation details in various programming languages. With the understanding of sliding window maximum algorithm, it will help to solve problems efficiently in different fields.