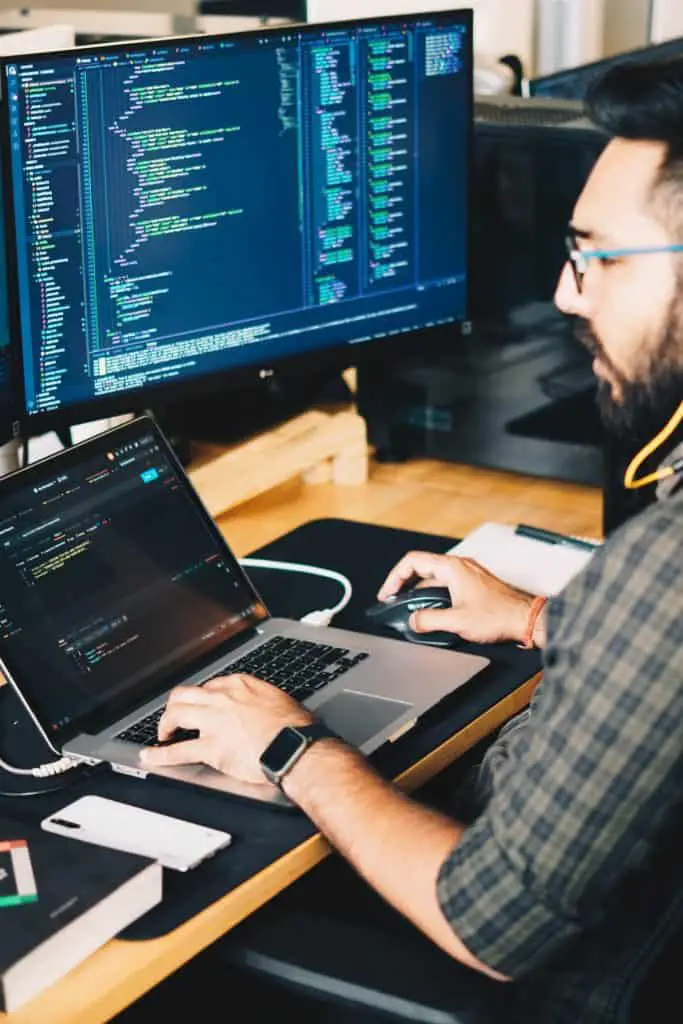
Before jumping straight ahead into ValueErrors, let us first take a moment to understand what an error is. Errors are problems in the program that disrupts the flow of execution. There are primarily three types of errors, namely: Syntax errors, logic errors, and exceptions. Today’s topic- Python ValueError.
ValueError falls into the category of these errors.
What are ValueErrors?
ValueError is raised by the Python program when the function receives a correct type but an invalid value.
‘Python ValueError: too many values to unpack (expected 2)’ occurs when the user is trying to access more or fewer values from the iterator than what was expected from the program.
To understand the above error in detail, let us start from the beginning.
- Firstly, functions in the python language can both accept and return multiple values and variables. So, when say, a function is assigned two values and during the execution of the program, the user enters only one value or enters more than two values, then a ValueError is raised.
- Secondly, this error usually occurs during unpacking, which is the assignment of iterable values in a tuple or a list.
To understand the concept in detail along with examples in various scenarios below is a detailed description with examples of the same.
Unpacking in list and tuples and ValueError solutions
This concept is better understood with examples. Below are the examples where the tuple unpacking and the ValueError are understood with examples and solutions.
The first case scenario is when the number of variables is greater than the number of values.
Example program 1:
print (“Enter the name:”)
firstname, lastname=input(“Enter a first and last name:”)
Output:
Enter the name:
Enter a first and last name: John Ray
—————————————————————————
ValueError Traceback (most recent call last)
<ipython-input-1-ff956b4283ab> in <module>
1 print (“Enter the name :”)
—-> 2 firstname, lastname=input(“Enter a first and last name:”)
ValueError: too many values to unpack (expected 2)
- Here, the program expects two values, but a single value is entered. Even if there is a space between first name and last name, it is still considered to be a single string. To solve the issue in this case, we can consider splitting the string in the place of the space between the first and last name.
- The same is corrected in the program below:
Corrected program:
print(“Enter the name:”)
firstname,lastname=input(“Enter a first and last name:”).split()
Output:
Enter the name:
Enter a first and last name: John Ray
Example program 2:
number1,number2=input(“Enter 2 numbers:”)
print(f”number1 is = {number1}\nnumber2 is = {number2}”)
Output
Enter 2 numbers:1,2
—————————————————————————
ValueError Traceback (most recent call last)
<ipython-input-14-d470e74671bf> in <module>
—-> 1 number1,number2=input(“Enter 2 numbers:”)
2 print(f”number1 is = {number1}\nnumber2 is = {number2}”)
ValueError: too many values to unpack (expected 2)
- Here, the program expects two values but a single value is entered, even if there is a comma between the first number and the next number, it is still considered to be a single value. To solve the issue in this case, we can consider splitting the value in the place of the comma between the first and next numbers.
Corrected program:
number1,number2=input(“Enter 2 numbers:”).split(“,”)
print(f”number1 is = {number1}\nnumber2 is = {number2}”)
Output:
Enter 2 numbers:1,2
number1 is = 1
number2 is = 2
- Now let us consider the opposite scenario where the number of variables is lesser than the number of values.
Example program:
print(“The list of first five numbers”)
element1,element2=[‘one’,’two’,’three’,’four’,’five’]
Output:
The list of the first five numbers
—————————————————————————
ValueError Traceback (most recent call last)
<ipython-input-5-71ca51d38e93> in <module>
1 print(“The list of first five numbers”)
—-> 2 element1,element2=[‘one’,‘two’,‘three’,‘four’,‘five’]
ValueError: too many values to unpack (expected 2)
- In general, the program usually works without any hindrance when the number of variables is equal to the number of values present and the above scenario can be rectified by unpacking using * or asterisk which stores multiple values without taking into account the length.
- The corrected program is given as:
print(“The list of first five numbers”)
element1,element2,*element3=[‘one’,’two’,’three’,’four’,’five’]
print(element1,element2,element3)
Output:
The list of first five numbers
one two [‘three’, ‘four’, ‘five’]
Example program 2:
print(“Enter your first, middle and last name:”)
element1,element2=[‘John’, ‘H’, ‘Doe’]
print(element1,element2)
Output:
Enter your first, middle, and last name:
—————————————————————————
ValueError Traceback (most recent call last)
<ipython-input-2-75dfd41790db> in <module>
1 print(“Enter your first, middle and last name:”)
—-> 2 element1,element2=[‘John’, ‘H’, ‘Doe’]
3 print(element1,element2)
ValueError: too many values to unpack (expected 2)
Here the scenario is that the number of values is much higher than the number of variables. It can be corrected using an underscore as a variable or * asterisk.
Corrected program:
Using underscore (‘_’)
print(“Enter your first, middle and last name:”)
element1,element2,_=[‘John’, ‘H’, ‘Doe’]
print(element1,element2,_)
Output:
Enter your first, middle and last name:
John H Doe
Using * asterisk
print(“Enter your first, middle and last name:”)
element1,element2,*element3=[‘John’, ‘H’, ‘Doe’]
print(element1,element2,element3)
Output:
Enter your first, middle, and last name:
John H [‘Doe’]
ValueError: too many values to unpack (expected 2) with dictionaries
In the Python programming language, a dictionary is a set of unordered items. The items in a dictionary are stored as a key-value pair. To start with, let us consider a dictionary named student_names. It consists of three keys, namely Student_1, Student_2, and Student_3. Each of the keys has a certain value stored against it. The values are what is on the right-hand side of the colon (:). If we were to print the dictionary items: keys and values individually, let us examine this scenario in the below code. Let us try to iterate over these dictionary values using a loop, in the below case a ‘for loop’. The idea is to print every single key and value pair separately. So let us now understand what happens if this code is run.
Example program 1:
data_entry = {‘Student_1’ : “Robert”,
‘Student_2’ : ‘Gerard’,
‘Student_3’ : ‘Albert’,
}
for keys, values in student_names:
print(keys,values)
The below output is observed.
Output:
—————————————————————————
ValueError Traceback (most recent call last)
<ipython-input-20-9f60c895b037> in <module>
—-> 1 for keys, values in student_names:
2 print(keys,values)
ValueError: too many values to unpack (expected 2)
There is a ‘ValueError: too many values to unpack (expected 2)’ error thrown on the execution of the above code. This happens because the program does not consider that both keys and values are separate entities in our ‘student_names’ dictionary.
To fix this error, we can use the items () function.
Now, what do items () function do exactly?
In the Python programming language, the items () method is used to return a list with all of the dictionary keys with values. Using this, we can now display the below output. It is printing the key and value pair.
Corrected program:
for keys, values in data_entry.items():
print(keys,values)
Output:
Student_1 Robert
Student_2 Gerard
Student_3 Albert
After observing the above scenarios, we can now both understand the meaning of the value error in lists, tuples, and also dictionaries and also solve them using simple replacements.
Example program 2
element_details = {
“name”: “Oxygen”,
“atomic_weight”: 15.999,
“atomic_number”: 8
}
for key, value in element_details:
print(“Key:”, key)
print(“Value:”, str(value))
Output:
—————————————————————————
ValueError Traceback (most recent call last)
<ipython-input-6-963247a1146f> in <module>
—-> 1 for key, value in element_details:
2 print(“Key:”, key)
3 print(“Value:”, str(value))
ValueError: too many values to unpack (expected 2)
Here, again the program can be corrected by using items() to iterate over the dictionary. The corrected program with the output is as follows:
Corrected program
element_details = {
“name”: “Oxygen”,
“atomic_weight”: 15.999,
“atomic_number”: 8
}
for key, value in element_details.items():
print(“Key:”, key)
print(“Value:”, str(value))
Output:
Key: name
Value: Oxygen
Key: atomic_weight
Value: 15.999
Key: atomic_number
Value: 8
Now to return the following dictionary in the form of a pair of keys and values, we can represent it in the form of tuples by using element_details.items(). It is as follows:
Program:
element_details = {
“name”: “Oxygen”,
“atomic_weight”: 15.999,
“atomic_number”: 8
}
print(element_details.items())
Output:
dict_items([(‘name’, ‘Oxygen’), (‘atomic_weight’, 15.999), (‘atomic_number’, 8)])
Other example of ValueError: too many values to unpack (expected 2)
def main():
print(“This program is used to compute the average of two examination scores.”)
marks1, marks2 = input(“Enter two scores separated by a comma: “)
average = (int(marks1) + int(marks2)) / 2.0
print(“The average of the scores is:”, average)
main()
Output:
This program is used to compute the average of two examination scores.
Enter two scores separated by a comma: 96,78
—————————————————————————
ValueError Traceback (most recent call last)
<ipython-input-12-eb04a09b4ba4> in <module>
7 print(“The average of the scores is:”, average)
8
—-> 9 main()
<ipython-input-12-eb04a09b4ba4> in main()
2 print(“This program is used to compute the average of two examination scores.”)
3
—-> 4 marks1, marks2 = input(“Enter two scores separated by a comma: “)
5 average = (int(marks1) + int(marks2)) / 2.0
6
ValueError: too many values to unpack (expected 2)
This can be solved using ast.
Corrected program
import ast
def main():
print(“This program is used to compute the average of two examination scores.”)
marks1, marks2 = ast.literal_eval(input(“Enter two scores separated by a comma: “))
average = (int(marks1) + int(marks2)) / 2.0
print(“The average of the scores is:”, average)
main()
Output;
This program is used to compute the average of two examination scores.
Enter two scores separated by a comma: 96,78
The average of the scores is: 87.0
Conclusion
Now let us summarize the solutions provided above:
- Match the number of variables with the list elements or values – The first way to correct a value error is to make sure that the number of assigned values and the number of variables are the same, to ensure seamless flow of the program.
- To use loops to iterate over all the elements one element at a time- By using loops to iterate over the elements, we can be assured that the number of elements and the variables are matched before execution.
- While we are trying to separate a key and value pair in a dictionary, use items()- This ensures that we have a list of all the keys and values and we can iterate over them without raising an error.
- We can store the multiple values while splitting the items into a list of elements instead of or separate variables
Frequently Asked Questions (FAQ)
1. What is the difference between a ‘TypeError’ and a ‘ValueError’?
A. A TypeError is present among many other Python exceptions. It is raised when an operation is performed on an unsupported data type or an incorrect data type. For example, when a function was expecting a string argument, but an integer was passed in its place.
But when we consider a ValueError, it occurs when the value that was to be entered is different from what was expected. Like for example, while unpacking a list, the number of variables that are entered is lesser than the actual length of the list.
2. What does ValueError: too many values to unpack (expected 2) mean in the case of a tuple?
A. The ValueError in the case of a tuple occurs when the number of elements in the tuple is more than or less than the number of variables that needed to be unpacked, then the above error is seen. The same is prevalent in the case of a list. When the number of elements in the tuple is less than or greater than the number of variables for unpacking, the above error occurs.
3. What are the easy ways to resolve the error ‘ValueError: too many values to unpack (expected 2)’?
A. This error can be resolved very easily, it can be done in the following ways:
- Being mindful of the number of variables and the number of values in the program. It is necessary to keep in mind that the number of values and the number of variables should be the same to ensure that an exception is not raised.
- Another way to ensure that the issue is resolved is, if in case the values are to be iterated in a list, then a loop like for loop can be used to ensure iteration and also using an underscore (‘_’) in place of a variable to store the value can also be done.
- In case the number of values is unknown, then an asterisk (‘*’) can be used to store all of those unassigned values in the form of a list.
- The above is applicable for tuples as well.
- In the case of dictionaries, when the above error is encountered, we can use .items (), to see the key and value pairs.