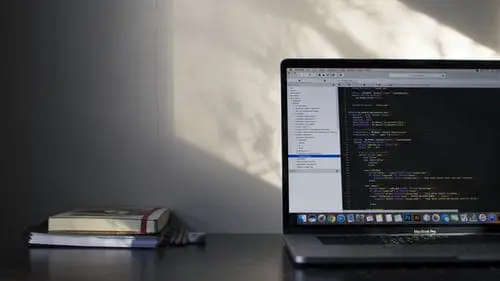
Linked List: A connected rundown is an assortment of hubs where every hub is associated with the following hub through a pointer.
What is the linked list?
A connected rundown is a powerful information structure where every component (called a hub) comprises two things, the information and a reference (or pointer), which focuses on the following hub. A connected rundown is an assortment of hubs where every hub is associated with the following hub through a pointer. This is one of the most simple and the commonest form of data collection. A connected rundown is a typical information structure made of a chain of hubs in which every hub contains a worth and a pointer to the following hub in the chain. The head pointer focuses on the primary hub, and the last component of the rundown focuses on the invalid. At the point when the rundown is vacant, the head pointer focuses on the invalid.
The types of the linked list
- Singly-Linked List
- Doubly Linked List
- What is a singly linked list?
The singly Linked rundown is a kind of information structure. In a separately connected rundown, every hub in the rundown stores the hub’s substance and a reference or pointer to the following node in the rundown. It doesn’t store any reference or pointer to the last node. A separately connected rundown is a kind of connected rundown that is unidirectional. That is, it very well may be crossed just a single way from head to the last hub (tail). Every component in a connected rundown is known as a hub. A solitary node contains information and a pointer to the following node, which helps keep up the construction of the rundown.
- How to reverse a singly Linked List using only two pointers?
It doesn’t look conceivable to turn around a basic, separately connected rundown. A basic independently connected rundown must be turned around in O(n) time utilizing recursive and iterative techniques. A memory effective doubly connected rundown with head and tail pointers can likewise be turned around in O(1) time by the trading head and tail pointers.
struct node {
int data;
struct node *link;
};
void reverse() {
struct node *p = first,
*q = NULL,
*r;
while (p != NULL) {
r = q;
q = p;
p = p->link;
q->link = r;
}
first = q;
}
We use the above-mentioned data to reverse the singly linked list by using only two pointers. You need two pointers, the first pointer, to pick the first hub. The second pointer to pick the second hub. Work out the time intricacy of the calculation you are utilizing now, and it ought to be clear that it can not be improved.
- What is the difference between Linear array and Linked List?
- Linear Array: A direct exhibit is a rundown of limited quantities of components put away in the memory. Components of the exhibit structure a succession or straight rundown. That can have a similar sort of information. Every component of the cluster is alluded to by a file set. Furthermore, the all-out number of components in the exhibit list is the length of a cluster.
- Linked list: Following are the different kinds of a connected rundown. Singly Linked List, item route is forward as it were. Doubly Linked List, items can be explored forward and in reverse. Roundabout Linked List, the Last thing contains the connection of the primary component. As next and the principal component has a connection to the last component as past.
- Linear Array: A direct exhibit is a rundown of limited quantities of components put away in the memory. Components of the exhibit structure a succession or straight rundown. That can have a similar sort of information. Every component of the cluster is alluded to by a file set. Furthermore, the all-out number of components in the exhibit list is the length of a cluster.
What are the applications of the linked list?
Application of the linked list in the science and technology is as follow:
- Usage of stacks and lines
- Execution of charts: Adjacency list portrayal of diagrams is most well known. Which is utilizes a connected rundown to store nearby vertices.
- Dynamic memory distribution, we utilize a connected rundown of free squares.
- Keeping up a registry of names
- Performing number juggling procedure on long numbers
- Control of polynomials by putting away constants in the hub of connected rundown
- Addressing inadequate lattices
Other applications of the linked list in the real world are:
- Picture watcher, previous and next pictures are connected, henceforth can be gotten to by the next and past button.
- Past and next page in the internet browser, we can get to past, and next URL looked in an internet browser by squeezing back and next button since, they are connected as a connected rundown.
- Music Player, songs in music players are connected to the past and next tune. You can play melodies either from the beginning or the finishing of the rundown.
- What does the dummy header in the linked list contain?
The dummy header in the connected rundown normally contains d first hub location, on the off chance that it is an ordinary single connected rundown. A fake head hub is a hub that consistently focuses on the start of the rundown or has a NULL pointer field if the rundown is unfilled. This hub is known as a fake hub since it doesn’t contain information remembered for the list. The first and last hub of a connected rundown typically is known as the head and tail of the rundown, separately. In this manner, we can navigate the rundown, beginning at the head and finish at the tail. The tail hub is an exceptional hub, where the following pointer is continually pointing or connecting to an invalid reference, demonstrating the finish of the rundown.
- How to add an item to the beginning of the linked list
- Make another hub, say newNode focuses on the recently made hub.
- Connection the recently made hub with the head hub. For example, the new node will presently highlight the head hub.
- Make the new hub as the head hub. For example, presently head hub will highlight newNode.
- To add a thing to the start of the rundown, you need to do the accompanying:
- Make another thing and set it worth.
- Connection the new thing to highlight the top of the rundown.
- Set the top of the rundown to be our new thing
If you are utilizing a capacity to do this activity, you need to adjust the head variable. To do this, you should pass a pointer to the pointer variable (a twofold pointer). so you will want to alter the actual pointer.
- What is a circular linked list?
The roundabout connected rundown is a sort of connected rundown. First thing first, the hub is a component of the rundown, and it has two sections: information and next. Information addresses the information put away in the hub, and next is the pointer that will highlight the next hub. The Head will highlight the rundown’s main component, and the tail will highlight the last component in the rundown. In the basic connected rundown, every hub will highlight their next component, and the tail will highlight invalid.
The round connected rundown is the assortment of hubs wherein the tail hub likewise points back to the head hub. The outline that appeared underneath portrays a roundabout connected rundown. Hub An addresses head, and hub D addresses tail. Along these lines, in this rundown, An is highlighting B, B is highlighting C, and C is highlighting D; however, what makes it round is that hub D is pointing back to hub A.
- How will you find the length of a given Linked List?
Here we will perceive how to discover the length of a connected rundown utilizing iterative and recursive methodologies. On the off chance that the head pointer is given, we need to follow these means to get the length.
- For iterative methodology − Take the top of the rundown. Until the current pointer isn’t invalid, go to the following hub and increment the tally.
- For recursive methodology − Pass head as a contention, the base condition is the point at which the contention is invalid. At that point return 0, in any case, recursively go into the rundown and send the following hub from the current hub, return 1 + length of the sublist.
- How can you detect a cycle in a linked list?
To eliminate the circle, we should get a pointer to the last hub of the circle. For instance, hub with esteem 5 in the above chart. When we have a pointer to the last hub, we can make this hub as NULL and circle are no more. A cycle happens when a hub next focuses back to a past hub in the rundown. The connected rundown is not, at this point, straight with a start and end—all things being equal, it goes through a circle of hubs. There is a cycle in a connected rundown if some hub in the rundown can be arrived at again by persistently following the following pointer. Inside, pos is utilized to signify the hub’s file that the tail’s next pointer is associated with. Track down the basic point on top of it by utilizing Floyd’s Cycle identification calculation. Store the pointer in a brief variable and keep a check = 0. Navigate the connected rundown until a similar hub is reached again and increment the check while moving to the next hub. Print the considered length of the circle.
Also read Is Python a Scripting Language or Programming Language?