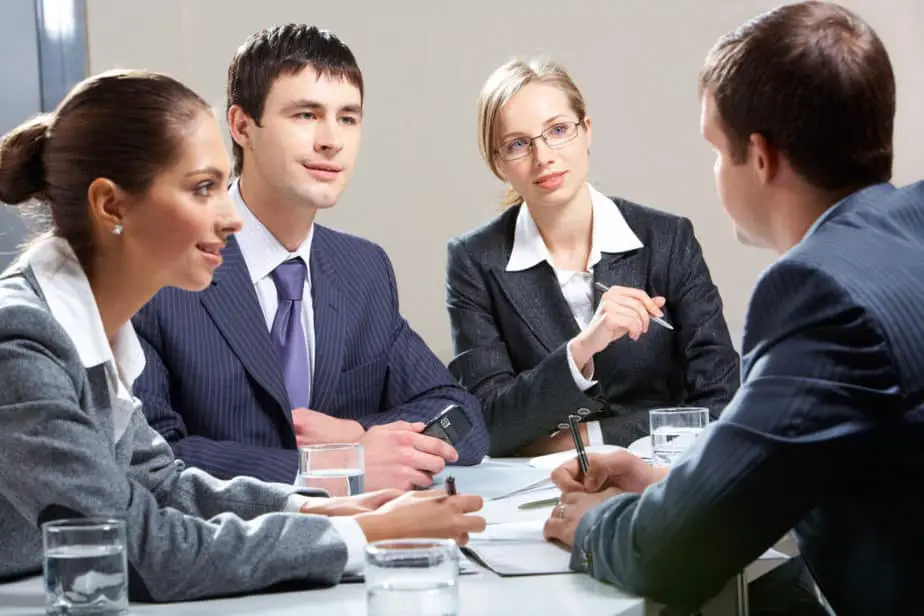
Introduction
Technical interviews focus on Object-oriented languages like Java, as it is used widely in industries these days. Java concurrency is an advanced topic the interviewer can ask you questions regarding this. Threading and concurrency are equally important from the job perspective. If java is the company’s core language, then they want the employee to be thoroughly skilled in java. Here, we’ll see some Java Concurrency Interview Questions.
What type of questions are asked in a Java interview?
Concurrency questions are essential during any java interview. In investment banking interviews concurrency and multithreading are asked and are tricky. It is difficult to write concurrent code. So, companies look for a well-experienced person in java.
Important Topics Under Concurrency
- Executors class
- Parallel Fork-Join framework
- ExecutorService class
- Callable and Future classes
- Executor class
Highly Asked Interview Questions
- What is the difference Between a Thread and a Process in Java?
When a program is in execution, it is called a process, i.e., the running program, whereas a thread is a portion of the process. The process has varied threads. We can consider processes as independent and do not share memory whereas, a thread is dependent. Time requires by process execution is more and threads require less time for execution.
- Explain what is Interrupting a Thread?
The interrupt method indicates the thread to stop the task it is currently working on. An exception ‘InterruptedException’ is thrown whenever the interrupt method is called, whether the thread is in a sleeping or waiting state, an exception is thrown. Flags have two modes true and false. When the thread is interrupted, the flag is set to true by the interrupt method ().
- What is JMM?
JMM is a Java memory model. In concurrent java applications, JMM designates how multiple threads access common memory. It also specifies how the changes are seen in other threads if changes are made in one of the threads. Internally it is divided into thread stack and heap. It also consumes OS memory.
- What are the Notify, Notifyall and Wait methods of the Object class?
Individual threads are independent, they wait, run, notifies and can be locked. These steps do not depend on or care about other threads. The wait method signals the current thread to release the lock and sleep or wait unless some other process enters and calls notify() or Notifyall(). Notify method wakes up the thread in sleep mode and Notifyall wakes up every thread paused on the object’s interface.
- Explain atomic classes in Java concurrency API?
Operations performed single-handedly are atomic operations. Some classes support atomic operations. All variables are counted as atomic except long and double. We use them in a multithreaded environment. Increment functions are not atomic as when one thread reads the value and increments it another thread enters and reads the older value, all this leads to wrong results. But using synchronization we can solve this problem.
- What is the lock interface in java concurrency API and state its benefits?
Synchronized methods enable efficient locking operations. They have a flexible structure. A thread has a lock only if no other thread holds it and is available. The lock interface has many explicit lock functions such as ReentrantLock, read-write lock, stamped lock, etc.
The benefits of the lock interface:
- We can set up a lock within time, acquire and take it off at any time.
- We can acquire and release locks in different scopes as well.
- Explain what is concurrent collection classes in java?
Concurrent collection class is part of the java.util.concurrent package. It’s an advancement of an existing collection of API. If collection is changed, when some thread is crossing over it is the iterator.next function will throw an ConcurrentModificationException. Few of the classes are ConcurrentHashMap, CopyOnWriteArrayList and CopyOnWriteArraySet.
- Explain Executors class in java?
The only class to support the callable implementation is executors. They can be used to create a thread pool in Java. Executor service in Java is the part that allows us to perform tasks on threads. Different executors are SingleThreadExecutor, CachedThreadPool,ScheduledExecutor,etc.
- Mention some main components of concurrency API?
The main components of java concurrency API are:
- Executor – It is an object on implementing execute tasks. Tasks don’t need to be asynchronous.
- Executorservice – It is an extension of the executer () method. It implements executor and after that a series of methods to execute threads. In this, a runnable is required to implement Executorservice.
- ScheduledExecutorService – It is similar to the Executorservice but different in terms of time. It performs tasks periodically. In this runnable and callable both methods are used to define the tasks.
- Future – A result is obtained after checking whether the operation is completed or not. Cancel function will cancel the task and if a flag isInterruptRunning states true the current task is terminated.
- CountDownLatch – It blocks threads while the process is completed.
- CyclicBarrier – It is the same as CountDownLatch only difference is it can be reused.
- Phase r- It is similar to and better than CounterDownLatch and is reusable.
- Lock – It is also used for blocking and lock and unlock are done in different methods.
- delayqueue – Delay queue is the priority queue and is based on the delay time.
- blockingqueue – It is a queue in which implementation depends on the queue is empty or blocked.
- threadfactory – It is an object that creates new threads.
- semaphore- It is used for blocking threads
- What is a Blocking queue?
Blockingqueue blocks the threads when they try to insert elements when the queue is full or remove elements when the queue is empty. A blocking queue functions like a queue it waits for the queue to get empty when values need to add. If we try to store a Null value a NullPointerException is thrown by blocking queue. Producer consumer problems can be solved using the java collections framework.
- What is callable and future in java?
The callable interface is similar to runnable, it belongs to the package java.util.concurrent.Callable, able to throw exceptions and return objects. Callable returns java.util.concurrent.For future objects, we can find out the status of a callable object. get method is used to wait for callable objects and thereby returns the result obtained and the cancel method cancels the callable task.
- How to handle ConcurrentModificationException?
Firstly, ConcurrentModificationException occurs when one tries to modify the object concurrently as it is not possible to modify a thread when some other thread is incrementing over it. In a multithreaded environment convert the list to an array, this works for small lists. ConcurrentHashMap and CopyOnWriteArrayList classes can be used if your JDK is higher than 1.5.
- What is Java.util.concurrent package at a higher level in java?
Java 1.5 and higher have this package. java.util.concurrent consists of different classes to create multithreaded applications. Concurrency means running various programs parallelly. Executors, timing, queues, concurrent collections are some of the main components of this package.
- What Are the Differences Between Executorservice and Executor Interfaces?
Both these interfaces come under Java.util.concurrent package. The executor is a simple class and can be executed singly. They accept runnable interfaces. ExecutorService is the extension of the Executor interface with multiple methods to handle and check concurrent tasks. The complex task includes futures.
- What is the difference between CountdownLatch and CyclicBarriar in Java?
Both of them have one thing in common that is both wait for the thread to complete its execution. The difference between CountdownLatch and CyclicBarriar is CountdownLatch cannot be used reused if the count is 0 and the latch is set to open. whereas CyclicBarrier can be reused as the barrier can be reset after it’s broken.
Conclusion
It is important to understand the concepts of java rather than mugging up a specific number of questions. Java multithreading and concurrency are essential materials for interviews. Some subtopics should be focused on to ace the interview. The above mentioned are just a set of questions read more to gain more.
FAQs
- What is the difference between wait and sleep?
Sleep method releases lock whereas wait will not. The sleep () method is declared or defined in the Thread class and the wait() method is defined in the Object class. The wait method needs a synchronized block but sleep does not require it. Sleep thread starts after a specific interval, whereas the wait method needs notify and notifyAll to start waiting for a thread.
- How can we execute 10 threads sequentially without using synchronization or any priority?
One way is to use lock method,
Reentrantlock l = new Reentrantlock();
l.lock();
// perform some operation
l.unlock();
- How do you prepare for a concurrency interview?
Understand the basics of concurrency and scheduling in the operating system. Through this one get to know what is semaphore, threads, deadlock, multitasking, etc. Secondly choose the language wisely, writing a code in C will be tedious than writing in ruby or go.
- What is a concurrency package in Java?
Java.util.concurrent is the concurrency package used in java. It consists of parallelism, multithreading, and concurrency on the Java platform. The backbone of concurrency is a thread.
- Is Java good for concurrency?
Java is still in practice and concurrency using java is effective. Java environment (JVM) provides libraries and that makes java different from others. Other languages like Python, Ruby, Go can also be used to implement concurrency.