Tree traversal is an important concept in computer science. It refers to the process of visiting each node in a tree structure in a specific order. There are two types of tree traversal techniques: depth-first search (DFS) and breadth-first search (BFS). DFS traversal of a tree using recursion is a powerful algorithm for traversing a tree and can be used to solve many problems. In this article, I’ll discuss the advantages and disadvantages of DFS traversal using recursion, as well as how to implement it practically.
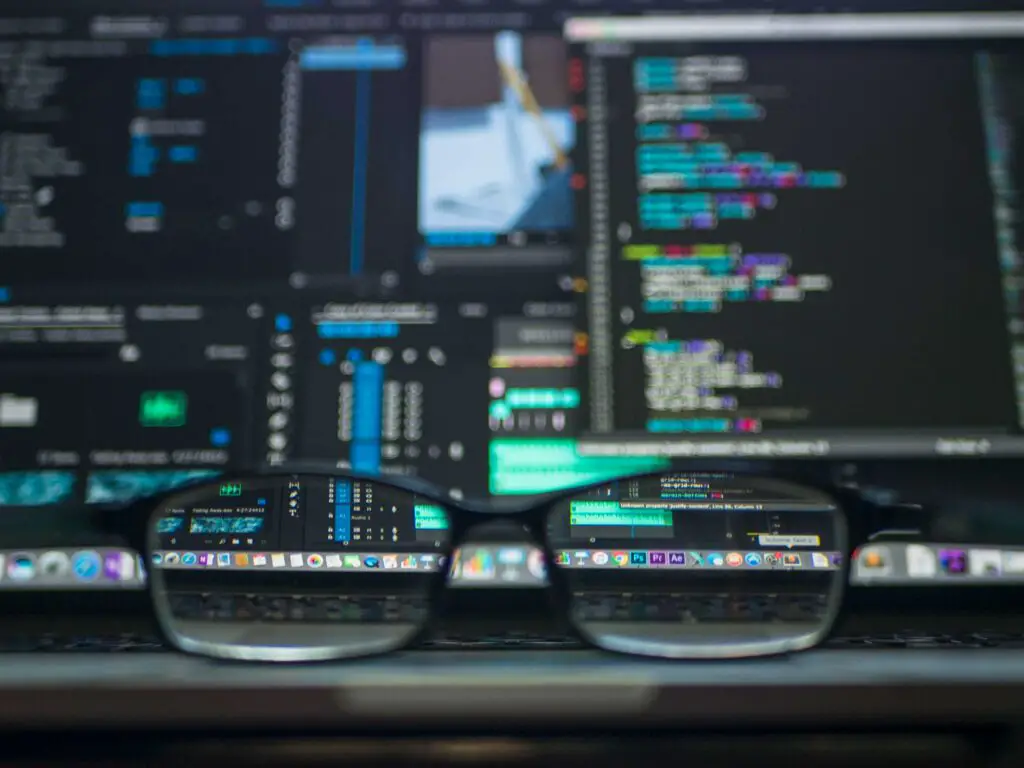
DFS Traversal Of A Tree Using Recursion
When implementing the DFS traversal of a tree using recursion, it is important to remember that each recursive call should return the results from each subtree before making the next recursive call. This ensures that each level of the tree is properly explored before proceeding down the other branch.
The Algorithm
Depth-first search (DFS) is a technical algorithm for traversing tree structures or graphs. It starts at the root node and widened as far as possible along each branch before backtracking. The idea is to go further and deeper into the tree before exploring other branches.
To traverse a tree in DFS, the idea is to start at the root node, explore each branch down the tree, and visit the nodes in each branch in a depth-first manner. This means that you first traverse all of the nodes on the left side of the tree, then all of the nodes on the right side of the tree, and so on.
To accomplish this, you will use recursion. In the case of DFS, each time the function is called, it will pass a different node to explore. The base case is when there are no more nodes to explore, and the traversal is complete.
Once all of the nodes have been visited, the order of their visitation can be used to construct a path from the root node to any other node in the tree. This path can then be used for various applications, such as finding the shortest path between two nodes or determining if two nodes are connected.
Furthermore, by storing the nodes in a data structure while they are being explored, you can determine which paths have already been explored, so they do not need to be re-explored.
The complexity of the DFS traversal depends on how much work needs to be done when visiting each node. For example, if the cost of visiting each node is constant, then the complexity would be O (V + E), where V indicates the number of vertices and E indicates the number of edges.
However, if additional processing needs to occur for each vertex during the visit, then the complexity would depend on how much work needs to be done during each vertex visit.
The Implementation
To implement the Depth-First Search (DFS) traversal of a tree using recursion, we need to understand three basic steps:
1. Identify the root node of the tree
2. Make Traverse the left subtree by recursively calling the DFS function
3. Make Traverse the right subtree by recursively calling the DFS function
For example, consider the following binary tree:
/ \ / \
4 5 6 7
The root node is 1. Then, we traverse its left subtree by recursively calling the DFS function with node two as the argument. The left subtree of 2 is 4 and 5, so we call the DFS function four and then 5.
Similarly, for node 3, we traverse its left subtree by calling the DFS function with node six as the argument. We continue this process until we reach the leaf nodes.
We can also use a stack data structure to store the visited nodes and use it to backtrack. This method is usually known as depth-limited search.
It is also important to have a track of which nodes have already been visited to avoid getting stuck in an infinite loop. By keeping these tips in mind, you should be able to successfully implement the DFS traversal of a tree using recursion.
A very useful application of DFS traversal is topological sorting. Topological sorting involves arranging elements such that all dependencies must come before their dependent elements. A topologically sorted list allows for efficient resource scheduling and workflow optimization.
The Time Complexity
When considering the time complexity of a DFS traversal of a tree using recursion, we must consider two distinct cases. In the best-case scenario, when the tree is balanced and all nodes are at the same depth, the time complexity is O(n). This is because all nodes must be visited at least once, so the total time complexity will be equal to the number of nodes in the tree.
However, in a worst-case scenario, such as an unbalanced tree, where some nodes are much deeper than others, the time complexity can become much higher. This is because, in this situation, some nodes may need to be visited multiple times to reach all other nodes in the tree. This means that the total time complexity could be as high as O(n^2).
To reduce the time complexity of a DFS traversal on an unbalanced tree, one possible solution is to use iterative deepening search (IDS). With IDS, instead of starting from the root node and exploring each branch until it reaches the bottom of the tree, it begins with a shallow search level and then gradually increases the search level until it has explored the entire tree.
This type of search has a much lower time complexity than regular recursive DFS since it only visits each node once. Additionally, it can help reduce the amount of memory used since only limited amounts of data need to be stored for each level of search.
Moreover, IDS can also help reduce backtracking since if a certain branch does not lead to any useful results, the algorithm can simply move on without having to start from the beginning again. As such, IDS can prove to be quite effective in reducing the overall time complexity of a DFS traversal on an unbalanced tree.
Conclusion:
In conclusion, Depth-First Search (DFS) traversal of a tree using recursion involves identifying the root node of the tree, traversing its left subtree by recursively calling the DFS function, and traversing its right subtree by recursively calling the DFS function. This process continues until all the nodes in the tree have been visited.
Additionally, a stack data structure can be used to store the visited nodes and backtrack.