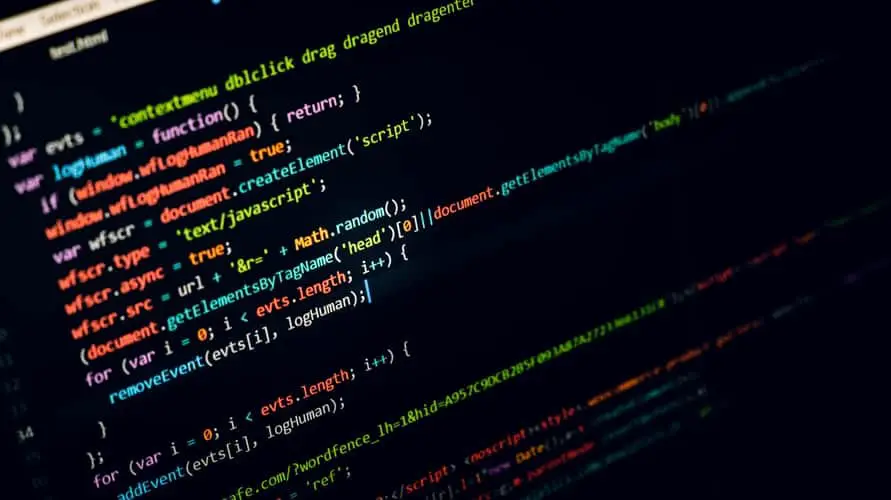
C# has been around for a while now. More than 50% of the top 500 companies in America use c#. Yes, C# is used in most companies, so recruitment and employment on c# frequent. But despite such wide usage, many people find it hard to secure employment in c#. This is mostly because most companies require candidates with advance s# expertise levels. In this article, I will ask a few popular questions that could be asked in C# Interview Questions for advanced developers. And then see the possible best answers for them. Join me as you increase your possibilities of crushing your next interview.
C# Interview Questions
Question 1: State The difference between Interfaces and abstract classes
Answer: There are several disparities between Abstract classes and interfaces. Let’s have a look at some popular differences.
- A class that is implementing a certain interface must provide implementations to all the methods provided by the interface.
- In rare cases, Abstract classes can be inherited without implementing the abstract methods. This would be in the case where the derived class itself is abstract.
- Interfaces usually have no state or implementation.
- Abstract classes can have implementations.
- Abstract classes cannot be multiply inherited. Meanwhile, for the sake of being able to implement multiple inheritances, the “Interface” was created.
Since interfaces and abstract classes serve similar functions and share similarities, here are some guidelines to help you know when to choose an abstract class over an interface or vice versa.
- Use an abstract class for declarations of sharable non-static or non-final fields.
- Use an abstract class for sharing codes between closely related classes with similar methods or fields.
- Use interfaces when you want to expose the properties or characteristics of a particular data type. But not just concrete enough to specify who will use it.
- Use interfaces when you want to take advantage of multiple inheritances of types.
Question 2: with short code snippets, explain the difference between overloading and overriding
Answer: Method Overloading is a programming concept that allows the creation of functions with the same name, under the same scope, but with different signatures. Below is an example that shows an overloaded method.
private static decimal DoAdd(int a, int b) {
return a + b;
}
private static decimal DoAdd(decimal a, decimal b){
return a + b;
}
Method Overriding is a programming concept that allows a child class to change the default functionality of an inherited method. Like that in the below example
public class Did
{
public virtual void FetchStuff(int id)
{
//Retrieve resources default location
}
}
public class Did2: Did
{
public override void FetchStuff(int id)
{
// base.FetchStuff(id);
// Retrieve resources new location
}
}
Question 3: If the below program is compiled, what will be its output, and why?
class Program
{
delegate void Printer();
static void Main(string[] args)
{
List<Printer> printers = new List<Printer>();
int i = 0;
for (; i < 10; i++)
{
printers.Add(delegate { Console.WriteLine(i); });
}
foreach (var printer in printers)
{
printer();
}
}
}
Answer: The above-written program will print out 10 ten times. This is because the delegate Printer() acts as a pointer, pointing to the variable i and not its values. Therefore, the value of i when the program gets to print is 10. The for-each loop’s body will execute printers.Count() times, and the content of printer() is Console.WriteLine(10), which will be printed printers.Count() times.
Question 4: Why is it wrong to specify access modifiers for methods interfaces?
Answer: On default, the access modifier for methods inside interfaces is public. This is because there is no other use for the methods() inside an interface except that a derived class could override it. Thus its reason for being public.
Question 5: What are the difference or similarities between these expressions below
Func<string, string> convertMethod = lambda; //A
public delegate string convertMethod(string value); //B
Answer: Statement A is a generic declaration of a delegate, then the declared delegate is assigned to a variable.
Statement B is just a definition of a delegate type. But both Statement A and B will be capable of holding the same method definition or lambda expression.
Question 6: Using code examples, explain anonymous types.
Answer: According to Microsoft Docs, Anonymous types enable the quick construction of objects with read-only properties without explicitly defining the object’s type first, especially for quick use inside a method.
var newData= new
{
FirstName = “Jignesh”,
SurName = “Trivedi”
};
Console.WriteLine(“First Name : ” + newData.FirstName);
In the above program, new data is an anonymous type.
Question 7: How do you make your data structure implement the where method and for each?
Answer: You can do this by making your data structure implement the IEnumerable interface
Question 8: Explain why the output of the program would change if you replace the ‘await Task.Delay(5); with Thread.Sleep(5). And what would be the first value before the change
Program:
private static string speak;
static void Main(string[] args)
{
Speaker();
Console.WriteLine(speak);
Console.ReadKey();
}
static async Task<string> Speaker()
{
await Task. (5);
//Thread.Sleep(5);
speak = “Hey Dude!”;
return “Say It Out Loud”;
}
Answer: await Task.Delay(5) asynchronously delays the Speaker() method for 5 milliseconds. This means That while the method’s execution is delayed, the program does not wait for it. It continues with other available tasks until the result is ready. While trying to continue with other things, the next task is printing to screen the string variable. Speak did not get to be initialized to “Hey Dude” because of the await (Making the program asynchronous). Before the 5 milliseconds get to be over( thus initializing the variable speak to “Hey Dude”), the program had already printed to screen an empty string. This is to say that when an async method encounters an await, it returns to the caller and continues execution until the result from the async method is needed, thereby eliminating the need to wait for results before they are needed. When the await Task.Delay(5) is changed to Thread. Sleep (5). The program will output “Hey Dude.” This is because an asynchronous method without an await keyword acts the same way a synchronous method would. And Thread. Sleep (5) only extends the execution time of the method by additional 5milliseconds.
Question 9: In c#, What function do using statement serve?
Answer: When a piece of code is wrapped with the using block, The using block ensures the disposal of whichever object was constructed just before the exit of the using block. The using statement implements the IDisposable interface on default.
Let us take an example from the code snippet below.
using (Resource myRes = new Resource())
{
myRes.DoSomething();
}
When the CLR( Common Language Runtime) comes across the above code, This is what it interprets;
{
Resource myRes = new Resource();
try
{
myRes.DoSomething();
}
finally
{
if (myRes != null)
((IDisposable)myRes).Dispose();
}
}
Question 10: Encapsulation, Explain how it is implemented in C#
Answer: When using access modifiers, you are in fact implementing encapsulation.
Access modifiers tell us how accessible a member of a class is from outside of the class.
- Protected: This access modifier allows a child class to access the base class’s member methods and fields. This is mostly used for inheritance.
- Private: This access modifier allows a class to hide its member methods and fields from external classes. It allows only methods inside of that class to access or use such members.
- Public: This access modifier allows a class to expose its members to external classes. Any member with public access modifier can be accessed from outside the class.
Question 11: Explain the concept of boxing and unboxing in C#.
Answer: C# was promoting a unified view of the type system, in which any value can be treated as an object. Boxing and unboxing make that possible.
- Boxing: This is a concept of converting a value data type to an object or interface that the value type can implement. So generally, by boxing a value, it means the CLR converts such value type to Object type by wrapping such object inside System. Object.
- Unboxing: This process is always done by code, explicitly. And it is a process of retrieving back the object converted to Object type back to its original value type.
Question 12: How does C# handle exceptions?
Answer: In C#, exception handling is done with the help of the below-mentioned keywords;
- Try: this block contains the code which will be checked for exceptions.
- Catch: This part of the program captures the error with the help of an exception handler.
- Throw: This throws an exception when there are problems in the code.
- Finally: This is used if you want to run a piece of code regardless of whether an error occurs or not
Question 11: What is managed and unmanaged code in C#?
Answer: Managed code is any code written with .net framework, or code developed by the framework or executed by the.net framework.
Unmanaged codes are codes developed outside the .net framework. Application not managed by the CLR is known to be unmanaged. Examples of unmanaged codes are those written in C and C++ to act as compatibility programs to access low-level system functionalities.
Also read Is Python a Scripting Language or Programming Language?