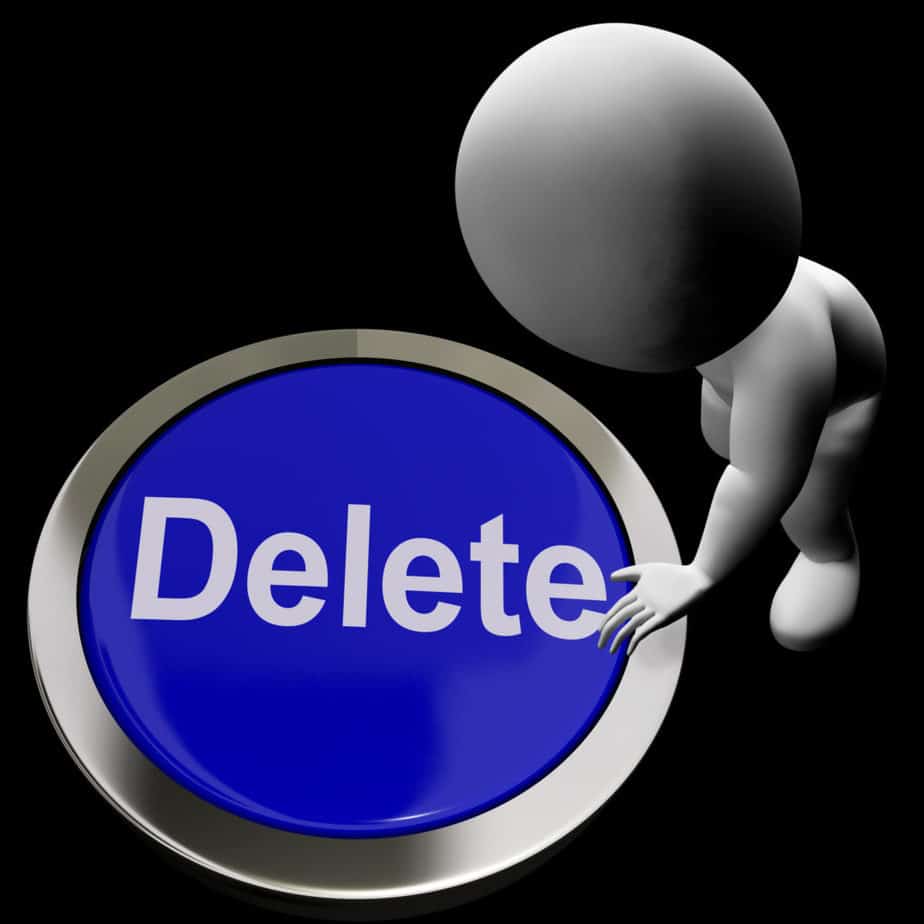
Writing a program for work? Or making your own mobile application or video game, there are several programming languages out there for you. Are you using Python? Great there are several versions and disciplines you can choose from. As of October 2019, there are 20 different versions of python available. What if you want to delete a file in python? You can delete the data on a file but what would be the need for an empty file, It only takes up more space.
Deleting can take various forms whether you want to delete a single file, an empty folder, a folder with multiple files in it, or files that have a specific ending. All your demands can be fulfilled using Python. All the deleting can be accomplished using 3 basic python methods: os.remove() method, os.rmdir() method, and shutil.rmtree() method.
The operations also require a few other modules that need to be imported to complete the operations all of which are discussed below. To delete any of the following using Python, just follow the simple steps given below:
1. To delete a file
2. To delete a folder
3. To delete files wild cards
4. To delete multiple
5. To delete a file in the folder with extensions
1. To delete a file
To delete just a single file the os. remove() method is used. However, documentaries cannot be deleted using it. Keep in mind that the os. remove() method can be used to remove only one file at a time. If you wish to remove multiple files at the same time you may use the shutil.rmtree() method which is used for more advanced operations.
Step 1
To use the os. remove() method, You need to first import it from the os library via Python import statement. Once you have imported the os module you can use it and are now only a few clicks away from deleting the file or files you wish to delete.
Step 2
After importing the os module, run the program. The simple code for deleting files is os.remove(file to be deleted). And click enter. On clicking the enter key there would be two possibilities. First, the program checks if the file even exists and if it does then deletes it. Two Python checks and after checking if it does not find the file, it then shows the message “file not found”. To save time and ensure the file gets deleted you can first check if the file exists and then delete it.
For Example:
The file to be deleted is “final_report”. So it should go something like this:
Import Os
Path: location leading to the file. “Home/project/final report”
If os.path.exists (“Home/project/docs/final report”)
os.remove(“Home/project/docs/final report”)
print (“final_report is removed”)
You can type just the name of the file instead of the Path, given that Python resides in the same folder then it automatically defaults to the current file, otherwise you need to input the file path.
2. To delete a folder
- For Deleting An Empty Folder
Deleting a folder is somewhat different from deleting a file. It requires you to import a different module. Required for this operation is os.rmdir() method. Keep in mind that this method can only be used to delete empty folders only. If the folder is not empty Python will display an error message. You can confirm the folder is empty using the os.listdir() method. It will show you a list of all the files that are present in the folder.
Step 1
After removing all the data from the folder, you can delete it using os.rmdir() method. For this first import Os.module if you haven’t already. After deleting the file “final_report”, Let’s say the empty folder that we wish to delete is “docs”. So the path would be “home/project/docs” the root leading to the final folder. You can also input the folder’s name directly given that Python resides in the same folder otherwise you are required to input the path.
Step 2
To ensure that the folder is removed we first check if the folder exists then check if it’s empty using os.listdir() and then use os.rmdir() to delete the empty folder. So you may use the following codes in order to delete this empty folder.
Import os
If os.path.exists (“home/project/docs”)
If len(os.listdir(“home/project/docs”)) == 0
Os.rmdir(“home/project/docs”)
Press enter after each sentence. You will then get either of the following texts.
Print(“home/project/docs is not empty”)
Print(“home/project/docs is removed”)
The first one is in case there is still some data present in the folder and the second one if the operation was successful.
- For deleting a folder with files
Let’s say we want to delete the folder “project’ which contains the file ”docs”. To delete a folder with all the data/files in it, you need to import from the Shutil library the Shutil module.
Step 1
Just like the os. remove() the shutil.rmtree()needs to be imported before it can be used.
Step 2
After importing shutil.rmtree() you are now ready to run the program. Use the following codes to delete a folder and all the files contained in it.
Import shutil
Shutil.rmtree(“home/project/docs”)
print(“home/project/docs is removed.”)
It is important to keep in mind that this function does not give a caveat before deleting the folder’s content so it is important to ensure all your important documents have been moved from the folder you wish to delete because once deleted the data cannot be recovered so make sure to double-check.
3. To delete files wild cards
For all the files in the folder with similar patterns, matching wild card patterns can also be used to delete files. To obtain all the files with a similar pattern we use glob. glob(pattern). We then repeat this for each of the files present in the folder. Each file is to be removed individually for this purpose you can use os. remove()method.
Step 1
First things first we import the globe module for our operation, To use it, we first need to import it. To obtain all the files with analogous patterns we use the glob.glob()method, entering our desired suffix in the brackets for example glob.glob(‘*.dxt’). Using this code in Python you will get a list of all the file paths that match this specific pattern.
Step 2
You may now use the os.remove() method to remove each file individually. It is pretty simple and very easy to follow. Let’s continue with our example of the suffix “dxt” mentioned earlier. Say we want to remove all the files with this pattern. The coding for this operation should go as follow
Import glob
Glob.glob(“*.dxt”)
os.remove(name of the file you want to remove)
This method is very easy and also helps save a lot of time. Instead of searching for all the files with the particular suffix, you can use the glob.glob()method, and then using the os.remove() method you can easily remove all the files you want to remove whilst keeping the ones you wish to keep.
4. To delete multiple files
You can easily remove a single file using the os.remove() method. What if you want to remove a long list of files well in that case you can use shutil.rmtree() method. It is very short and simple and will do all your work in just a few minutes.
Step 1
First, select all the files you wish to delete and move them to a separate new folder. Make sure to double-check all the files so that you do not lose any important data. Let’s say we have made the folder moved all the files we want to delete and named it “hoop”
Step 2
Once you have separated all the files that you wish to delete you may now import the shutil model from the library so that you can use it.
Step 3
After importing the shuttle model we may now use the shutil.rmtree() method to delete the folder “hoop” containing all the files we wish to delete. The simple coding for this easy operation is as follow
Import shutil
Shutil.rmtree(“hoop”)
print(“hoop is removed.”)
Following the above code, you will be able to delete all the files at once. Keep in mind that the shutil model is for advanced operations involving multiple files. Files once deleted cannot be recovered so make sure to check multiple times to ensure no important file is placed mistakenly in the folder to be deleted.
5. To delete a file in the folder with extensions
If you want to remove all the files with a specific suffix, ending, or extension in a specific folder you may use the following method to complete this operation. After choosing the particular extension we want to remove, all we have to do is input the path and the extension and python will do the rest.
Suppose we want to delete all the files that end with the extension “dxt”. And there are a bunch of these in our folder ”literature”. Supposedly the path folder is “home/notes/literature” and our folder “literature” contains the following files
• Pic2000.dxt
• Pic3000.dxt
• Pic4000.dxt
• Pic5000.dxt
• Img009045
• Pic6000.dxt
• Img006543
• Img098308
And we only wish to delete the files that end with the extension “dxt”. The programs that need to be imported to complete the operation are os.listdir() and the os module. It will be using os.listdir() to get a list of all the files that are present in the folder and will then show us all those files. Following are the steps you need to undertake in order to complete the operation.
Step 1
First, you need to import the os module and the os.listdir() from the library, as they will be required for completing the operation.
Step 2
After importing the programs now you need to specify the “file_path” . This is a road leading to folder leading to the folder which consists of all the files that you wish to delete. Continuing with the same example our “file_path” would be “home/notes/literature” leading us to the notes folder which consists of all the files.
Step 3
Now to delete the files with a specific extension “dxt” in this case we input the command that deletes files that end with the extension “dxt” using the os.remove() method. So first the program searches through the list provided by os.lisrdir() for the files with the required extension. After searching and finding all the files it acts upon the os.remove() command. The program should run something like this:
Import os
From os Import os.listdir
Folder_path: “home/notes/literature”
For file_name in listdir(“home/notes/literature”)
if file_name.ends with (‘.dxt’)
os.remove (“home/notes/literature”+ ‘.dxt’)
In places of “home/notes/literature” you need to add the location or path of your folder and instead of “dxt” you need to input your desired extension that you wish to delete.
Modules and methods used
• Os module
Used to interact with the operating system as it enables a portable process when using the operating system. The os in the os module stands for “operating system”. The Os module is a part of the two hundred Python’s Standard Utility Modules. In case of a wrong code, the os module would show an error message also known as the error
• Os.remove() method
The os.remove() method is a part of the Os module and is often imported when deleting files. The os.remove() method is used to delete one file at a time. It is mostly used to delete a single file. Directories and folders cannot be deleted using the os.remove()method. In case of incorrect code, the method would lead to os error.
• os.rmdir()method
The os.rdmir() method is another one of the methods that come under the os module. You can use the os.rmdir() method once you have imported os. It is used to delete empty folders and directories. folders It is to be noted that folders and directories containing data cannot be deleted using os.rmdir() method. If the folders or directories commanded to be deleted are not empty it would lead to an os error. To ensure that the folder or directory is empty you can always use the os.listdir() method. In case of incorrect code, the method would lead to os error.
• shutil.rmtree()method
The shutil.remtree is used for advanced operations that involves multiple files such as copying, removing, or deleting files. Whatever operation you plan to undertake you need to import os and then import shutil because the shutil.rmtree() is part of another library, You cannot use it without importing it. When removing a folder using the shutil.rmtree() method you need to provide a ”path” to the folder or documentary that you wish to delete.
A path is simply the road or a guide leading to the folder or directory that needs to be deleted. However, a symbolic link to the desired target will not work and lead to error. . In case of incorrect code, the method would lead to os error. Almost all copying and deleting can be accomplished using the shutil.remtree() method but keep in mind that metadata of files cannot be copied using this method. Another thing to take caution for is that folders and directories once deleted using the shutil.remtree() method cannot be recovered so make sure to double-check all the folders before deleting.
• os.listdir() method
Often used when deleting multiple files the os.listdir() method is a part of the os library and serves the function of providing a list of all the files present in a folder or directory. To see the files present in a folder or directory you need to provide a “path” to that particular folder or directory. “path” simply means directions leading to that particular folder that you wish to delete. If you do not provide a “path” to a particular folder or directory os.listdir() defaults to the current folder or directory and shows a list of all the files present in it.
• Glob module
The glob module comes under Python’s standard library and needs to be imported in order to be used. Whenever you wish to acquire files that have a specific extension you may use the glob module. For example, You want a list of all the files that end with the “dxt” you may then input that specific pattern in the glob module and it will present to you a list of all the files that have that particular extension. The glob module is very quick and believed to be faster than other programs used for the same purpose.
Python is one of the most frequently used programming languages. It has about 20 different versions. Python offers a variety of operations with over 200 different modules in its Python Standard Library almost all of your demands or operations can be completed by pairing appropriate methods. In order to use a module, you need to first import it from the library.
As deleting can take various forms Python all provides a variety of methods paired with certain modules to help accomplish your goals. For deleting a single file you can use the os.remove() method. If you want to delete multiple files, a folder or directories, or files with a specific pattern you can use the shutil.rmtree() method. For deleting empty folders you can use the os.rmdir() method. A little caveat here keeps in mind that files, folders or directories once deleted cannot be recovered so make sure to double-check all the files before clicking the enter key.